This article kicks off a series on building a Serverless API in Go in AWS using Lambda, API Gateway, and AWS Cognito! In this one, we’ll start by creating a simple lambda function using AWS’s tooling for Go.
Before you can follow this, you should have a basic understanding of Go, as well as an AWS account. I’ll be using VSCode on a Windows workstation, but you can very easily replicate this on Mac or Linux. Lets dive in!
Your First Go Lambda Function
Lambda is an AWS Service that allows you to write and execute serverless functions. Along with Go, it supports most of the popular development languages out there. It is essentially the backbone of any serverless offering thats hosted on AWS.
Lambda Functions may seem daunting to build and write, but once you understand the basic requirements, they become very easy to put together and deploy! Let’s write a simple Hello World Lambda and deploy it.
In VSCode, open an empty folder on your machine. Then open the integrated terminal and create a new Go module with the following command.
go mod init hello-world-lambda
You should have a single file named go.mod.
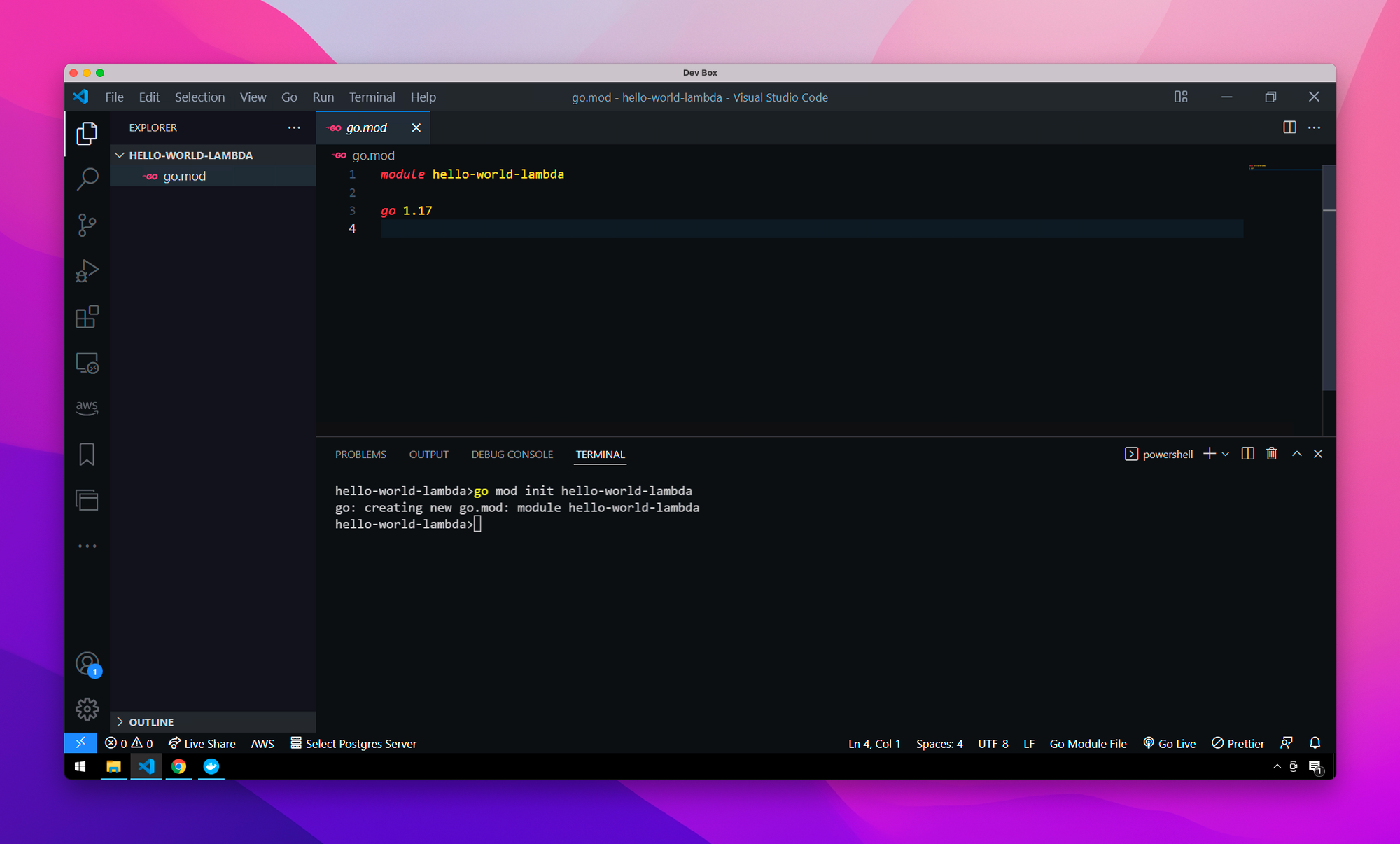
Now create your entry main.go file and populate it with the main function as usual. For good measure, add a log.Println method as well just to make sure we’re setup properly.
package main
import "log"
func main() {
log.Println("hello world!")
}
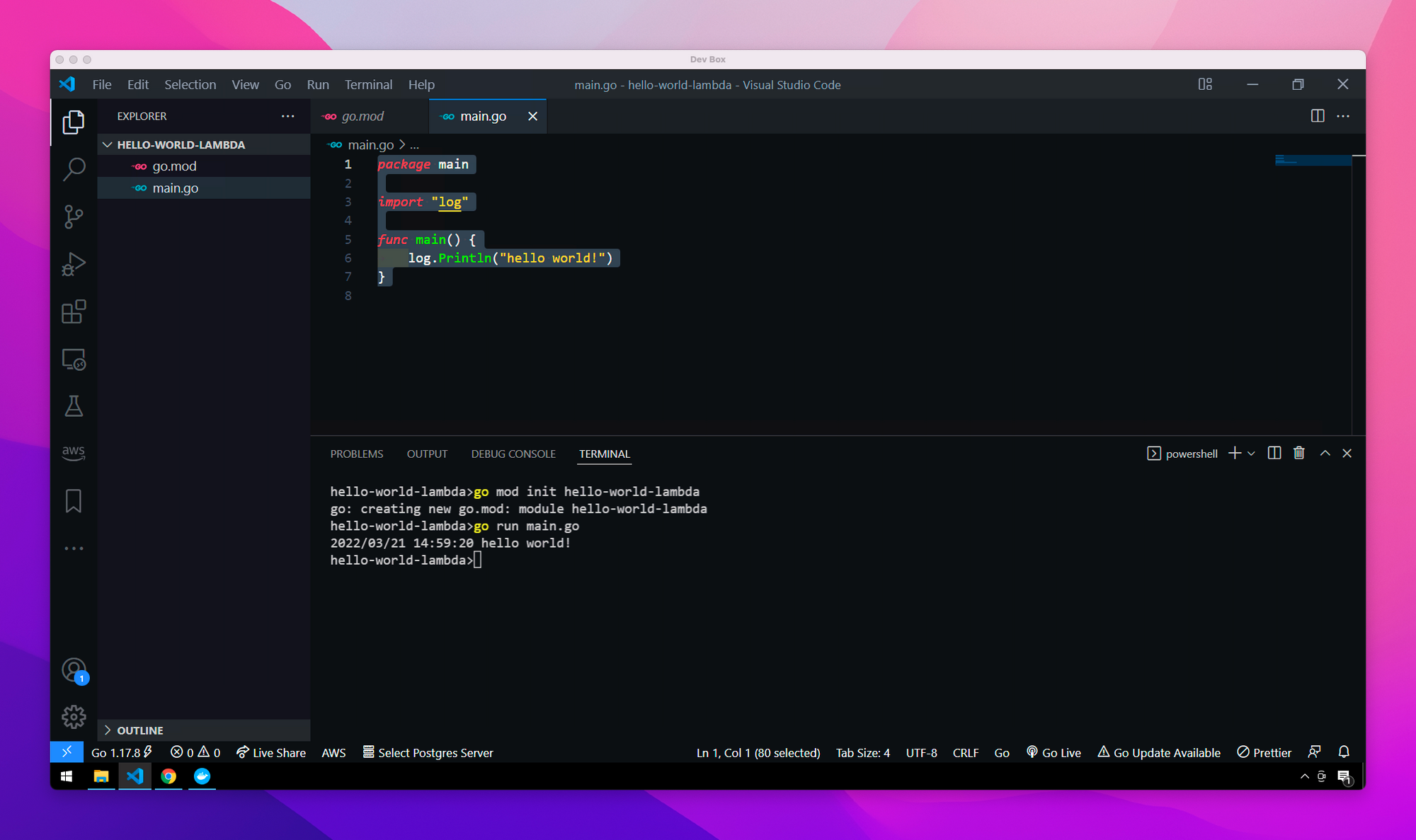
Before we continue, make sure you have the aws-go-sdk installed by running the following command in the terminal.
go get -u github.com/aws/aws-lambda-go
Now lets modify this to support Lambda, specifically the API Gateway Proxy Event which will be sent to our Lambda Function by API Gateway when we get to that portion of the series. Modify your code to match the following. Much of the code has been commented to describe what each section is doing. The vast majority of Lambda functions you find will generally start with this as a base.
package main
import (
"log"
// Importing our AWS Libraries
"github.com/aws/aws-lambda-go/events"
"github.com/aws/aws-lambda-go/lambda"
)
func main() {
// lambda.Start is how Lambda handles passing various events into your code
lambda.Start(handler)
}
// 'handler' will change the inputs & outputs depending on the type of event you trying to handle
func handler(request events.APIGatewayProxyRequest) (events.APIGatewayProxyResponse, error) {
// Logging a simple message to the console, which will display in the AWS Console
log.Println("hello world!")
// Creating our response with a status code of 200
response := events.APIGatewayProxyResponse{
StatusCode: 200,
}
// Finally, return the response with a nil error object to satisfy the outputs
return response, nil
}
Deploying the Lambda Function
To test, we’re actually going to package up our function and deploy it straight to AWS. From the AWS console, search for Lambda in the search bar and select it.
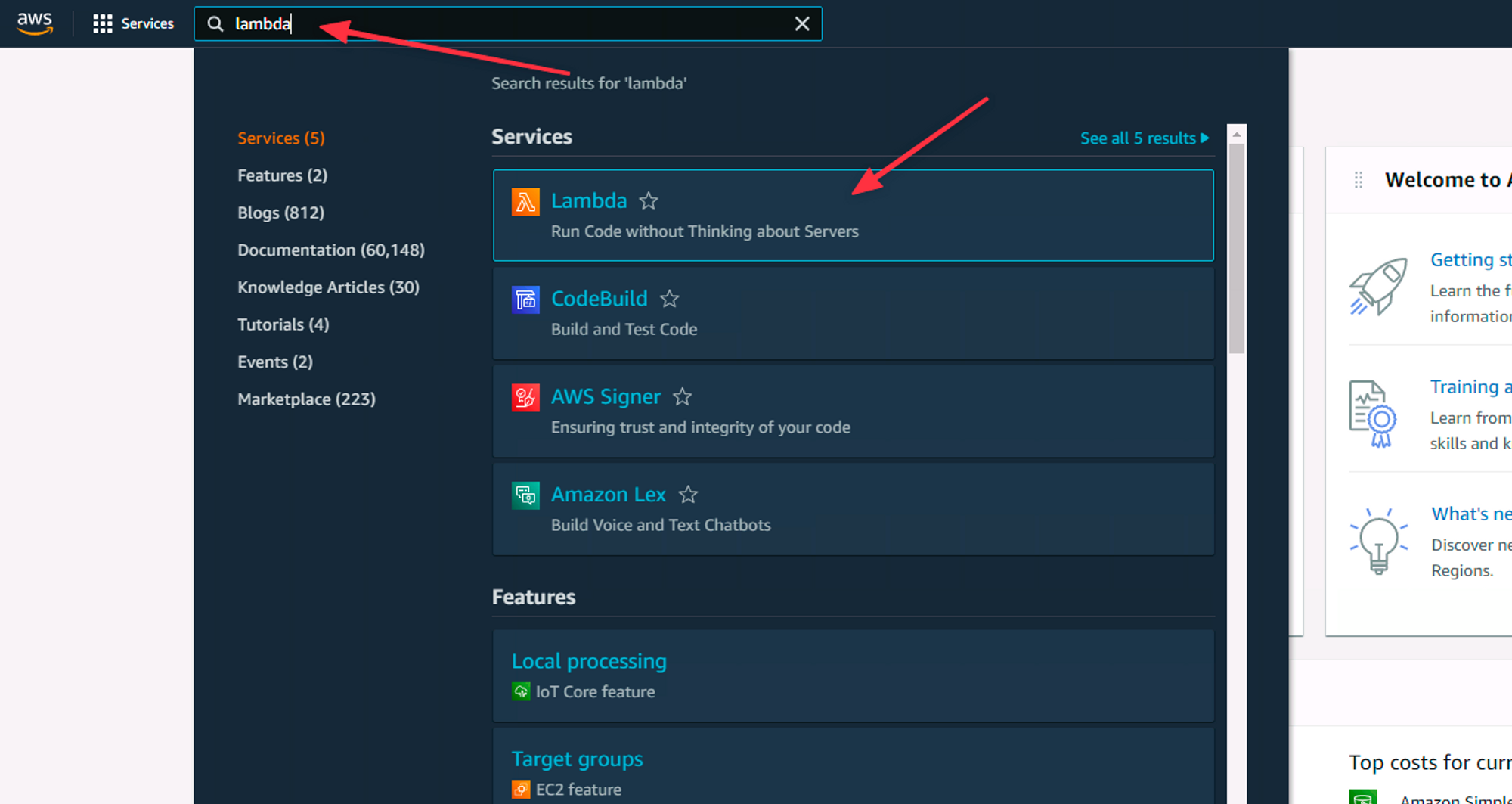
Once there, click on Create Function. Give your function a name and make sure to change the runtime to Go 1.x.
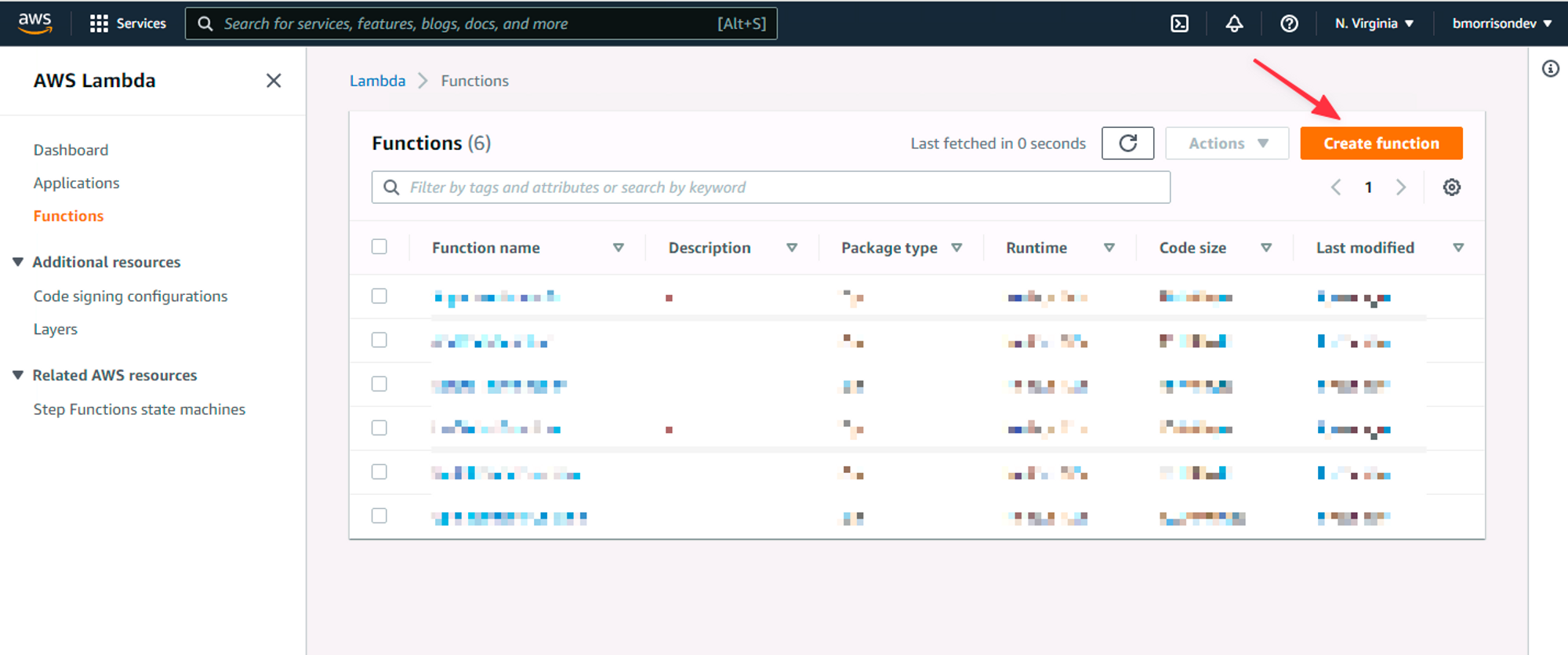
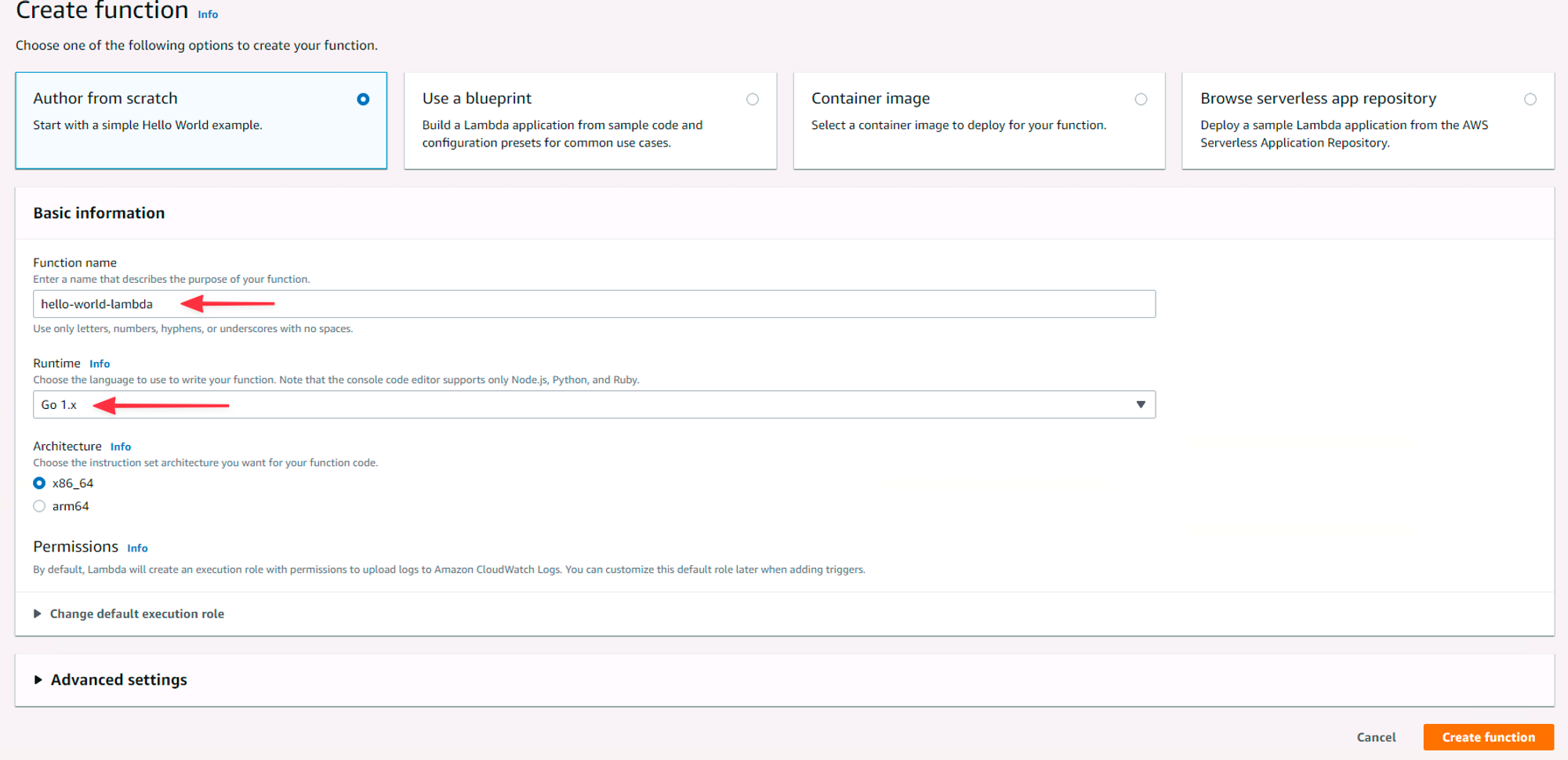
Now since AWS runs these on Linux, we need to specify the system we are building for with Go instead of just accepting the default. Run the following in your terminal to build the function.
# Run this on Windows
$Env:GOOS = "linux"
go build main.go
# Run this on Mac
GOOS=linux go build main.go
You should get a single file added to your file system called main. This is the compiled version of our Lambda function. You’ll need to zip up the file before you can upload it. On Windows, you can right click the file, open the Send To menu, and select Compressed folder
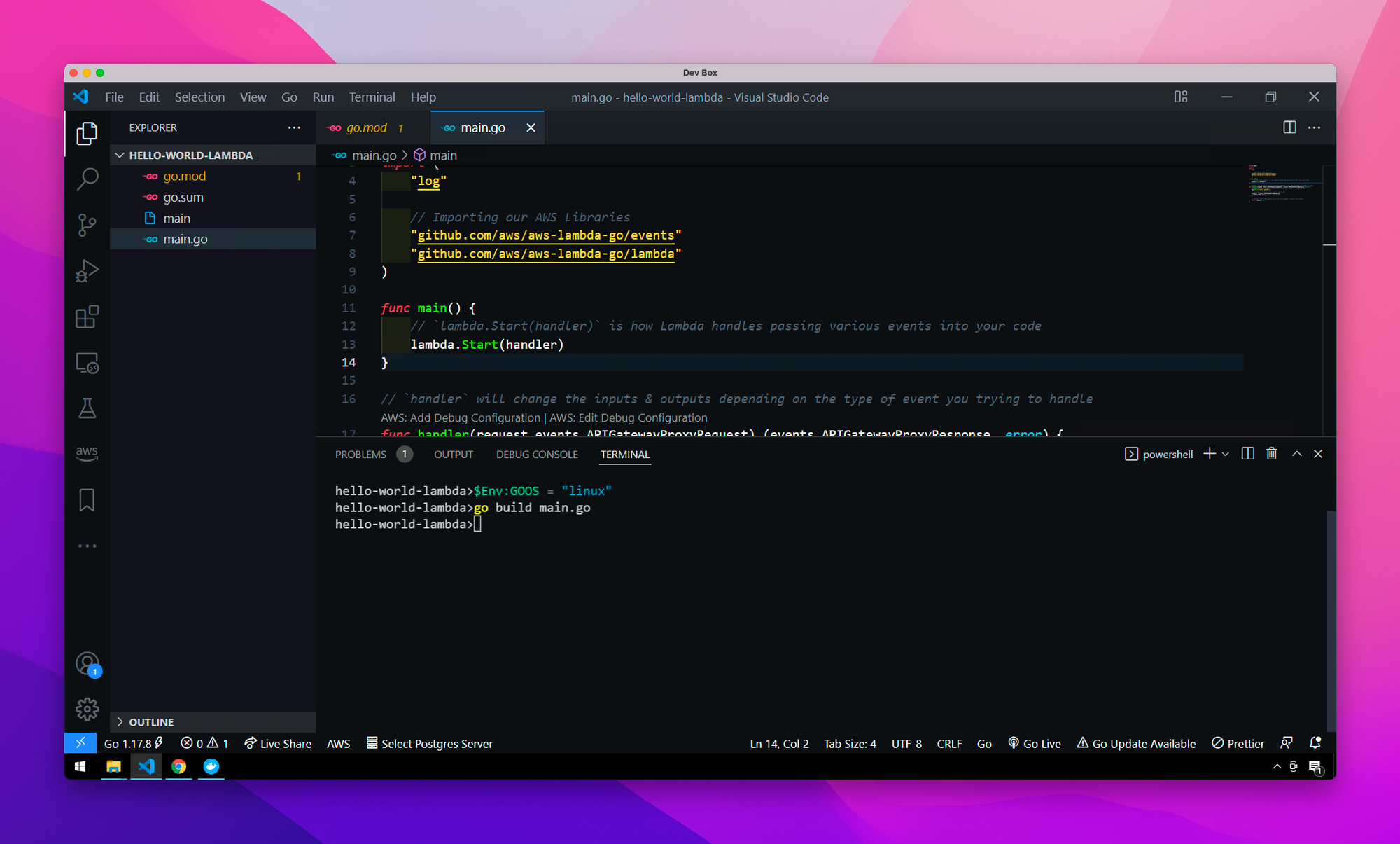
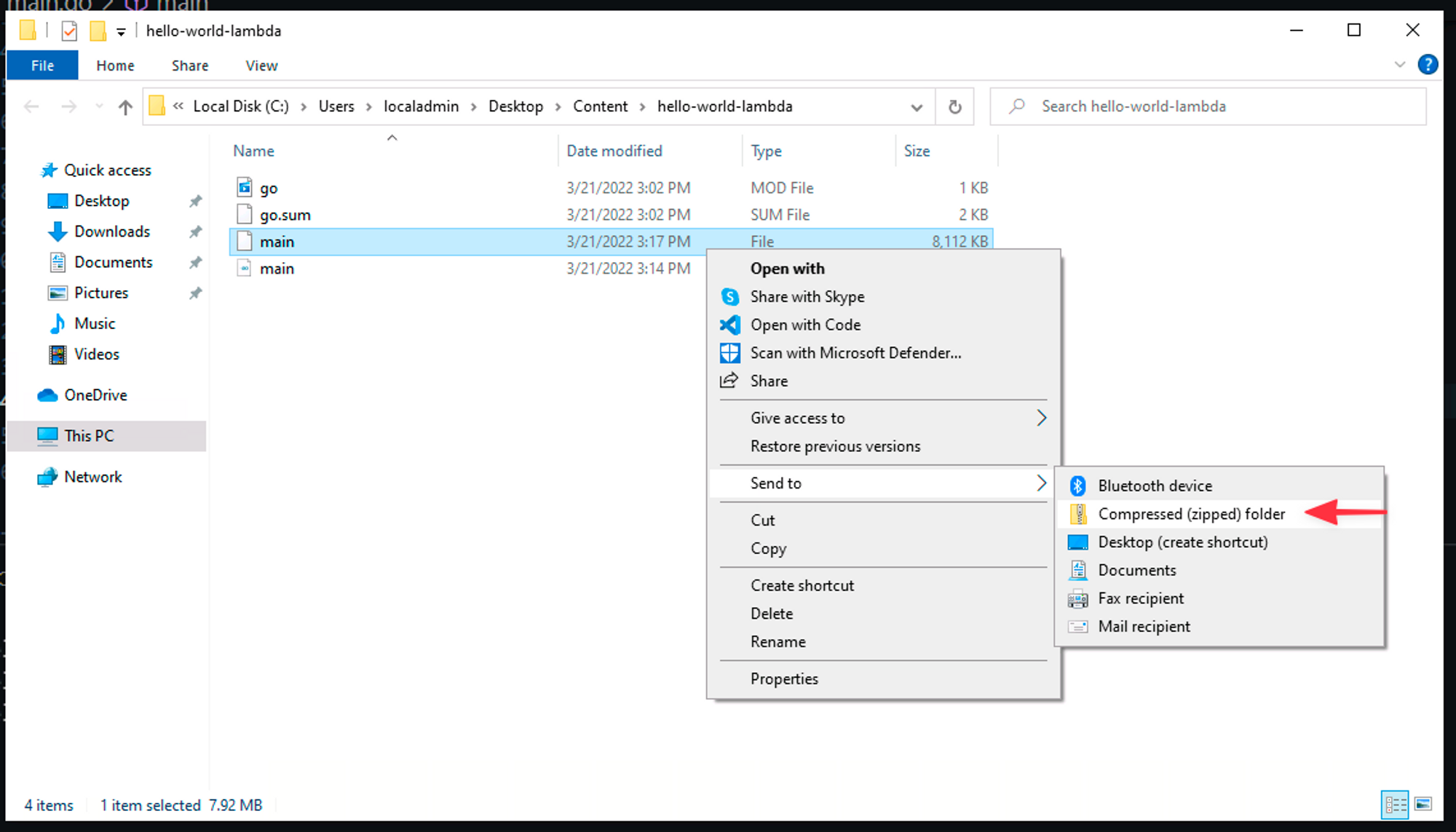
You should have a main.zip that was created. Now back in AWS, click the Upload From button, select .zip file. Select the zip you just created.
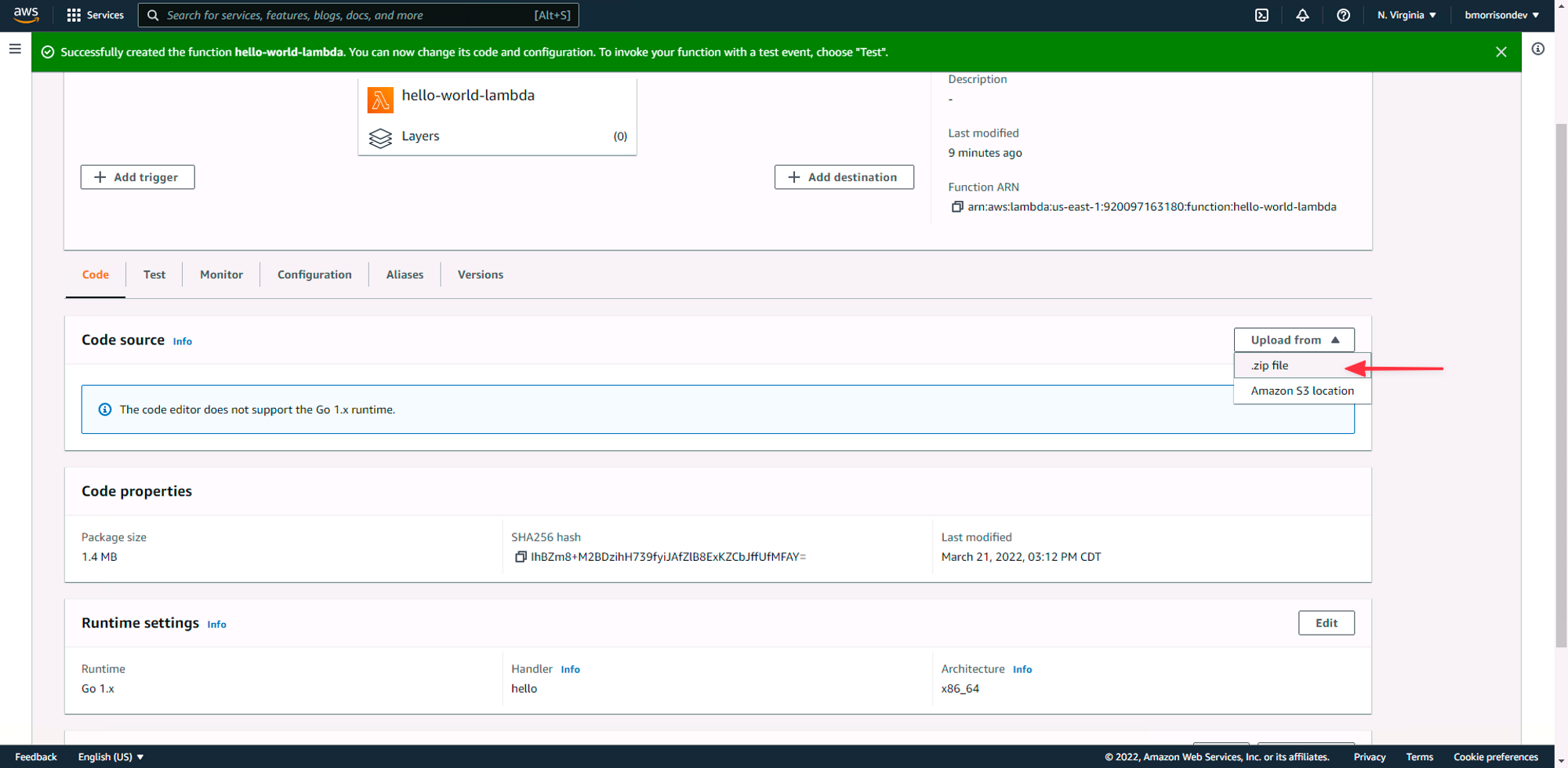
Once its uploaded, you’ll need to change the Handler in the settings from hello to main. Click on Edit in the Runtime Settings section, then change the Handler to main in the next dialog and save it.
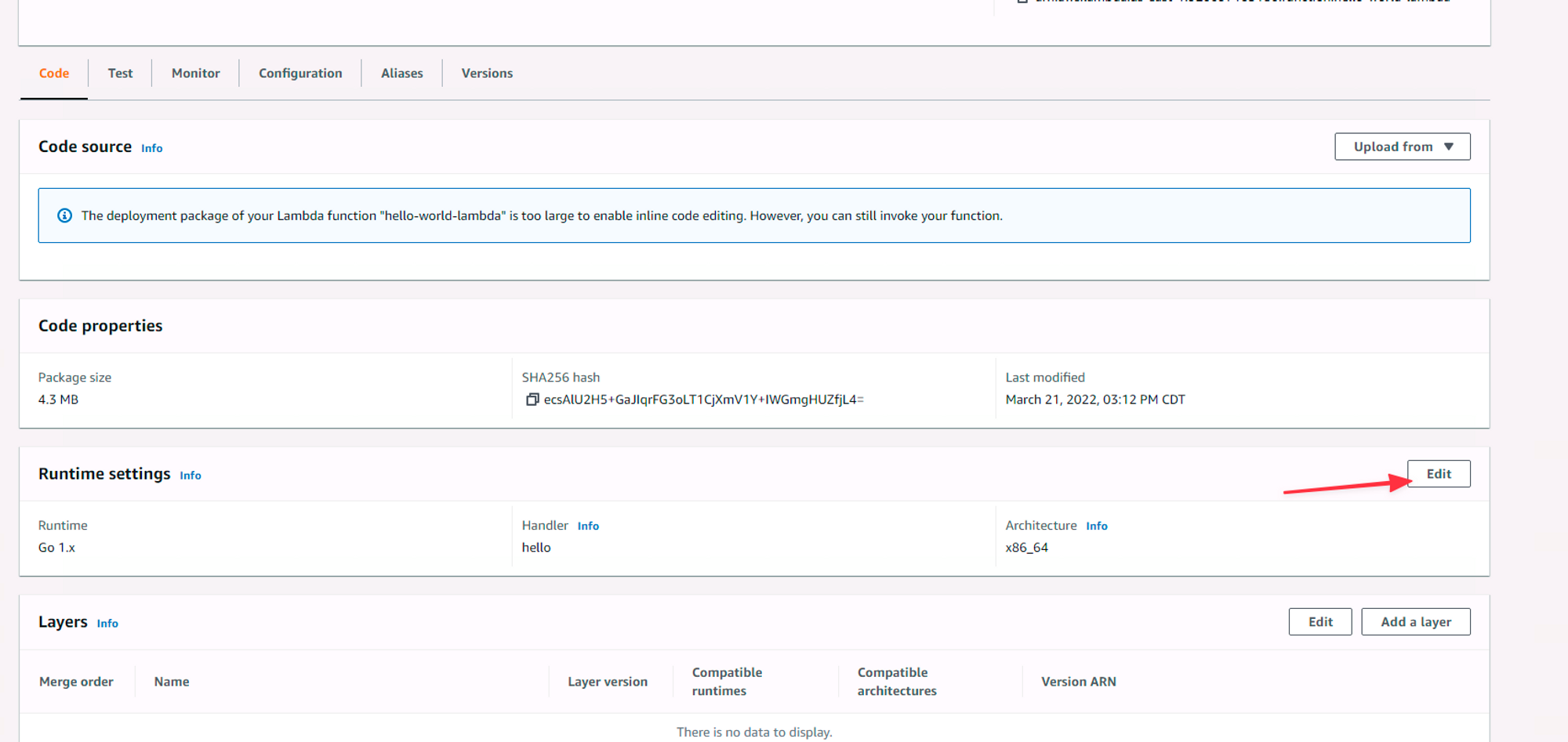
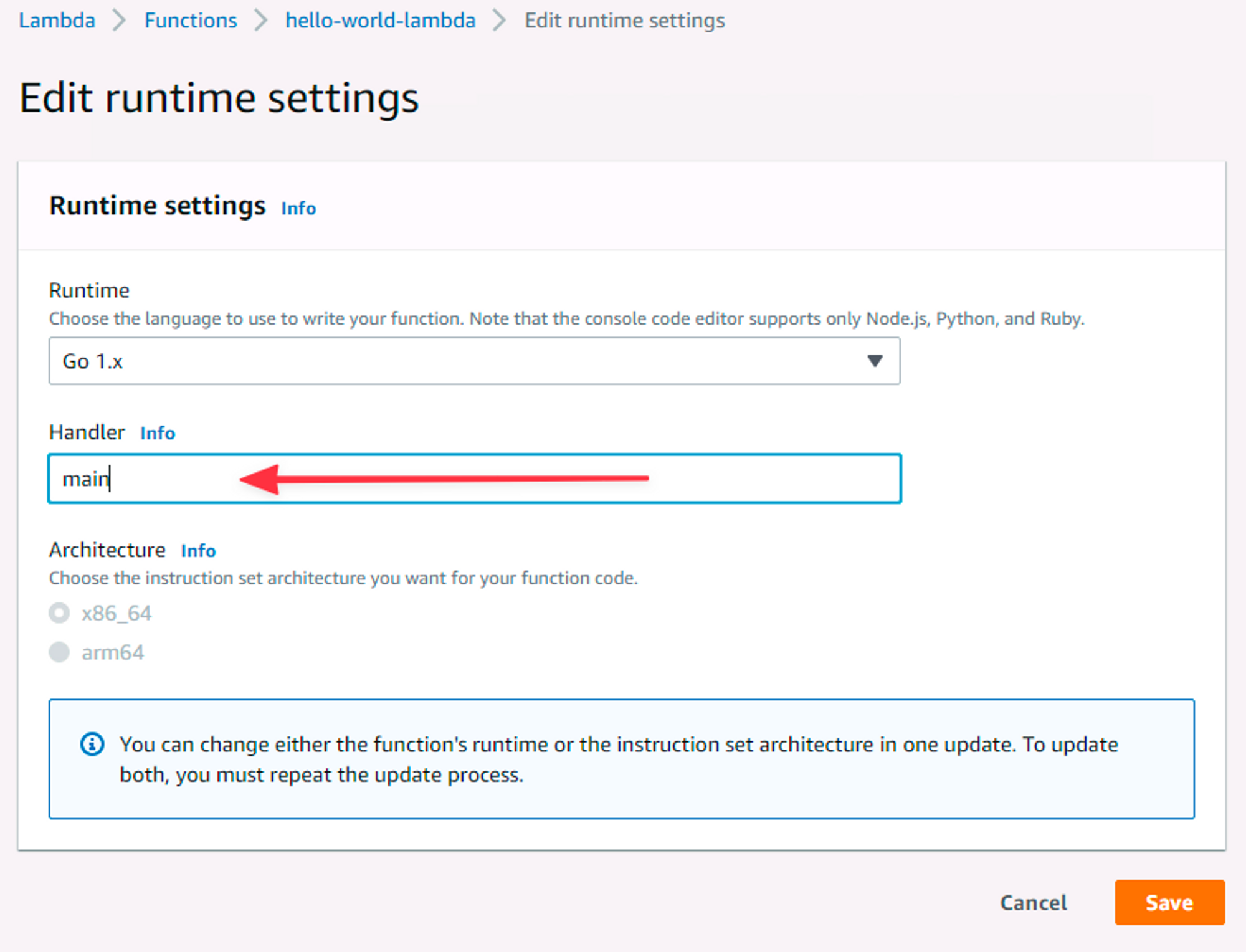
Testing Our Work
Now we can test the function. Head to the Test tab and simply click Test. You should have a green alert that shows up. Expand that to see the details of the execution. You can see we’ve returned that 200 status code, as well as see our hello-world
in the logs below!
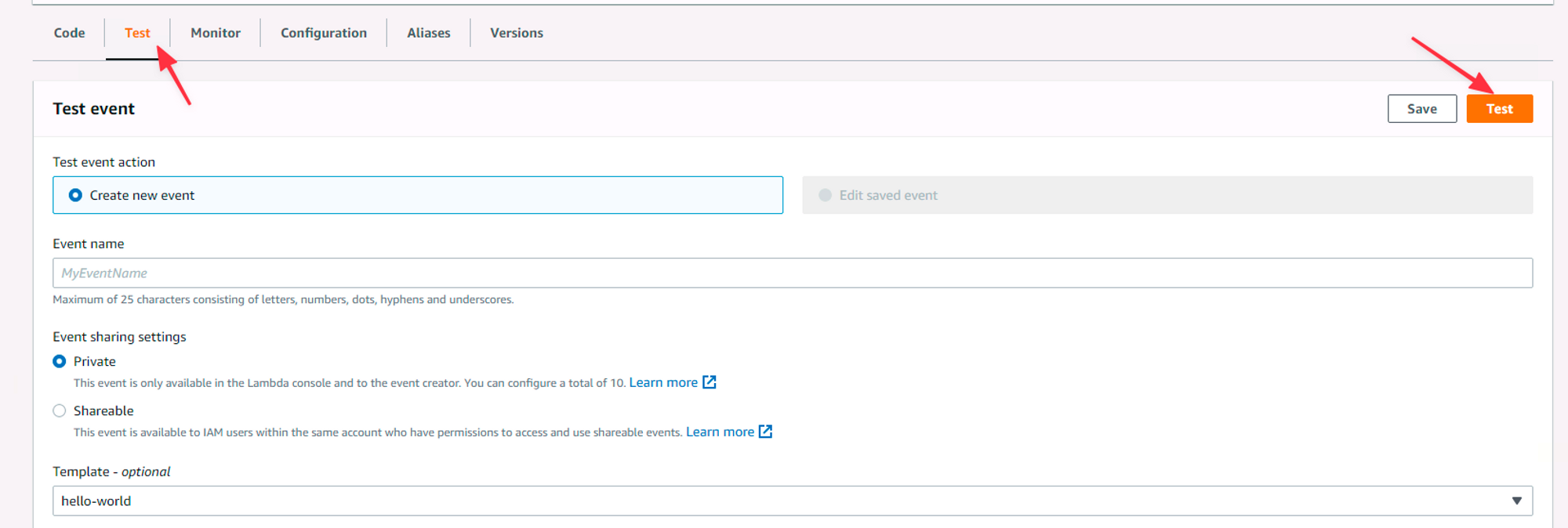
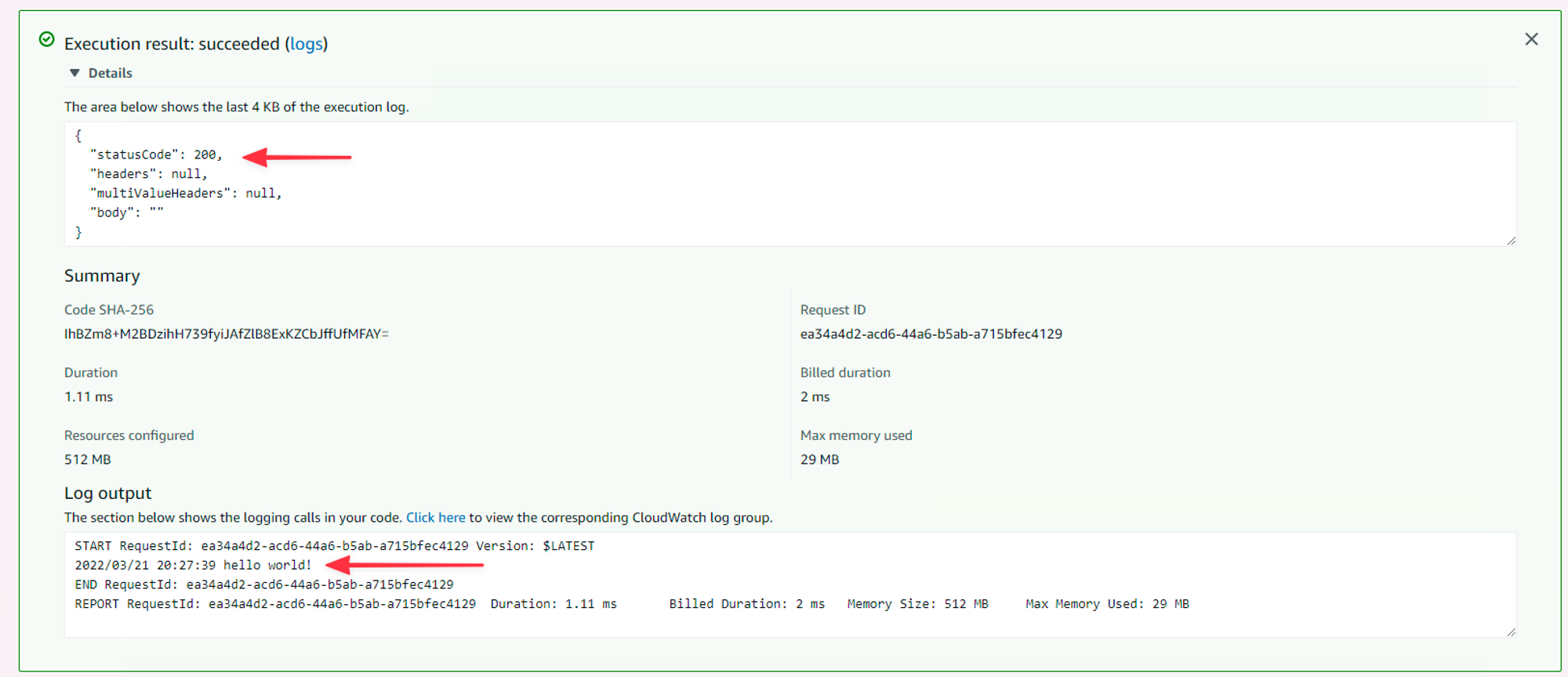
In the next entry, I’ll show you how to wire up an API Gateway to Lambda functions and hit our functions using Postman!