Now that you have a basic bot built, we’ll want to explore the different ways you can respond using the bot.
Direct Messages
Bots will parse any message they have access to, this actually includes direct messages to the bot itself. If you want to setup direct messages, you’ll actually need the bot to message you first. In this example, we’ll use the command !dm
to get the bot to message you to start. Add the following code into your message handler.
if(msg.content.startsWith("!dm")) {
let messageContent = msg.content.replace("!dm", "")
msg.member.send(messageContent)
}
Your index.js should look like this now;
// Import discord.js and create the client
const Discord = require('discord.js')
const client = new Discord.Client();
// Register an event so that when the bot is ready, it will log a messsage to the terminal
client.on('ready', () => {
console.log(`Logged in as ${client.user.tag}!`);
})
// Register an event to handle incoming messages
client.on('message', async msg => {
// This block will prevent the bot from responding to itself and other bots
if(msg.author.bot) {
return
}
// Check if the message starts with '!hello' and respond with 'world!' if it does.
if(msg.content.startsWith("!hello")) {
msg.reply("world!")
}
if(msg.content.startsWith("!dm")) {
let messageContent = msg.content.replace("!dm", "")
msg.member.send(messageContent)
}
})
// client.login logs the bot in and sets it up for use. You'll enter your token here.
client.login('your_token_here');
Start your bot if it isn’t already (or restart it), and issue the !dm
command we just added. You should receive a direct message from the bot.
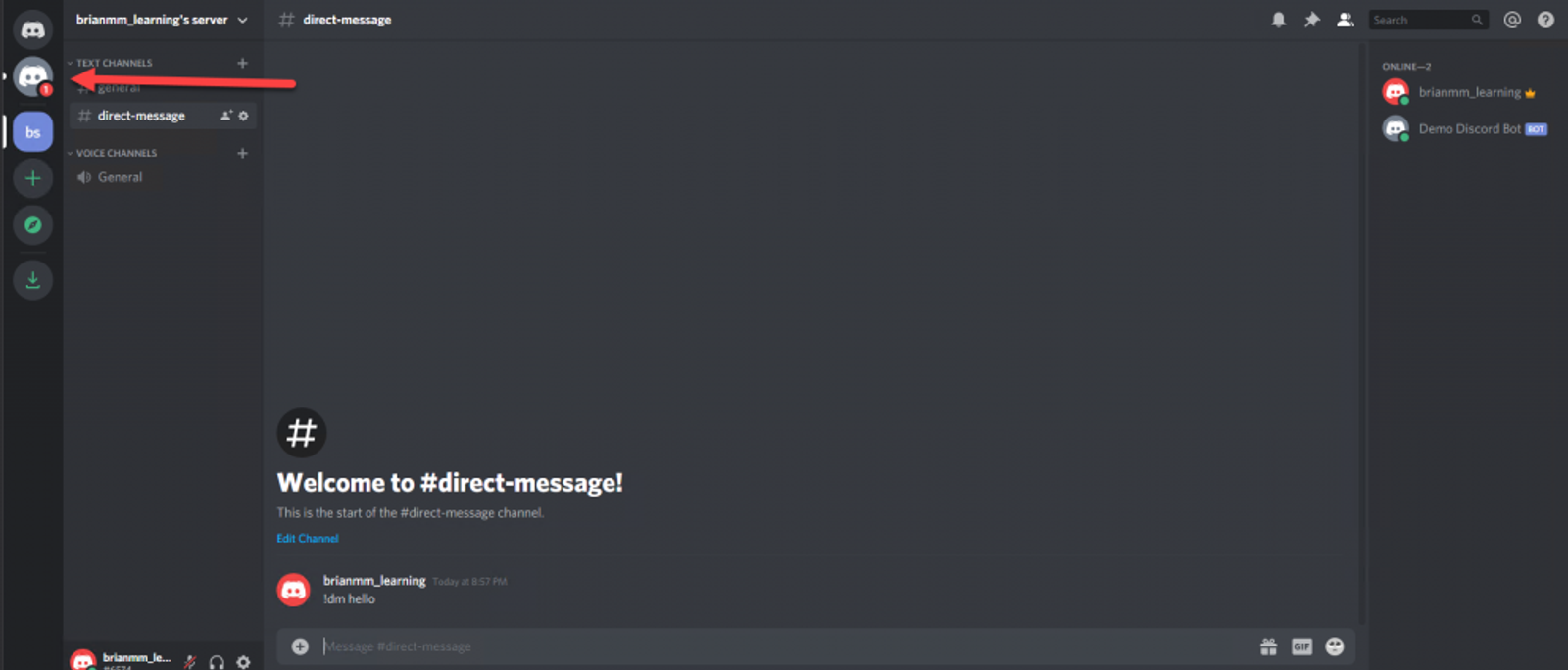
Any command we’ve setup the bot to handle so far can now be issued using the new chat. If you’re coming from my last post, you can issue the !hello
command and it should respond in the chat.
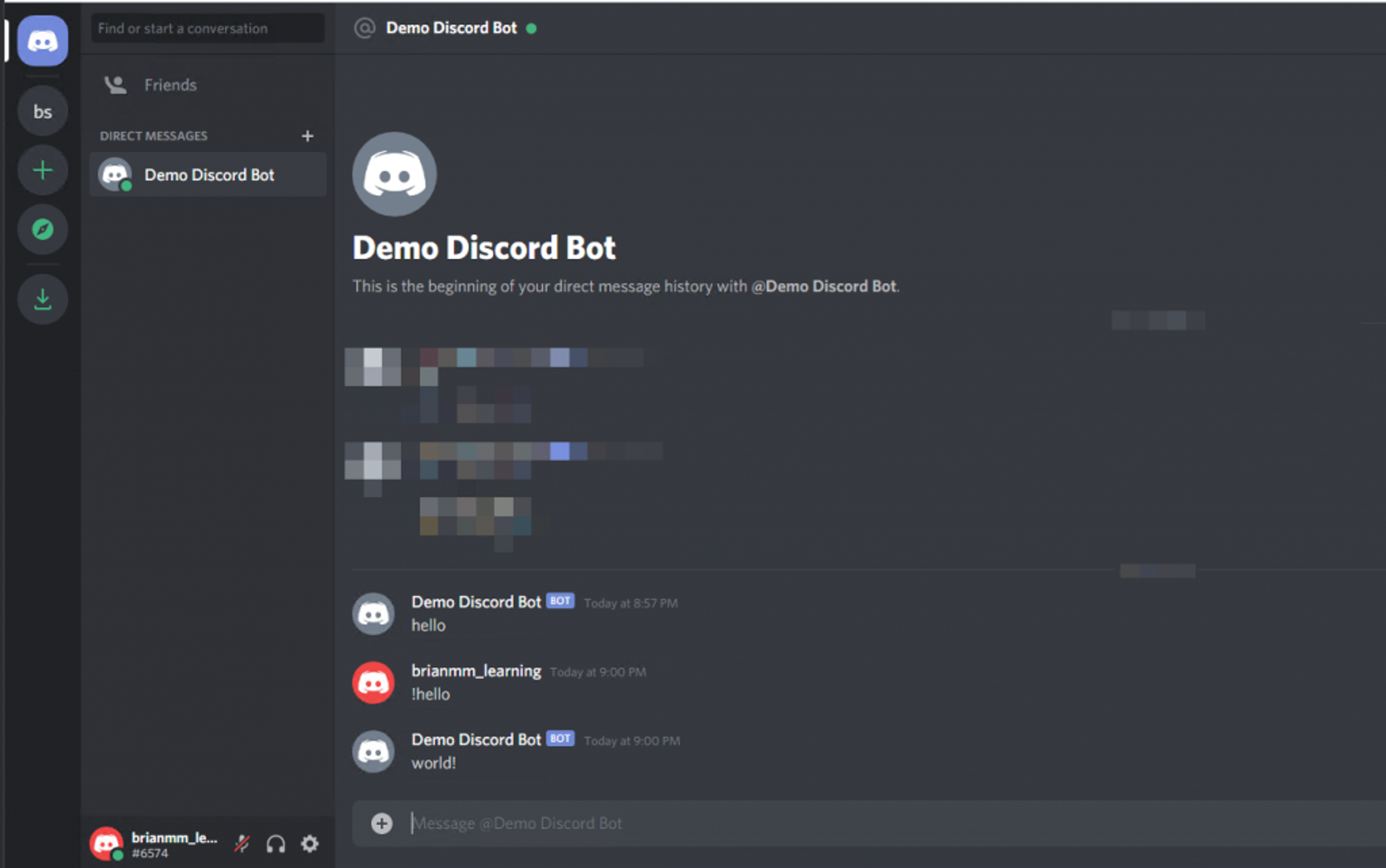
Processing Arguments
So far, we’ve been using very basic commands to get our bot to respond, but you can also parse out the entire content of the message sent and handle it differently based on the full input. Let’s add some additional code to parse the message to respond differently, even if the command prefix is the same.
if (msg.content.startsWith("!args")) {
const args = msg.content.split(' ')
let messageContent = ""
if (args.includes("foo")) {
messageContent += "bar "
}
if (args.includes("bar")) {
messageContent += "baz "
}
if (args.includes("baz")) {
messageContent += "foo "
}
msg.reply(messageContent)
}
Now if we issue the !args
command with a different message to follow, our bot will respond in different ways.
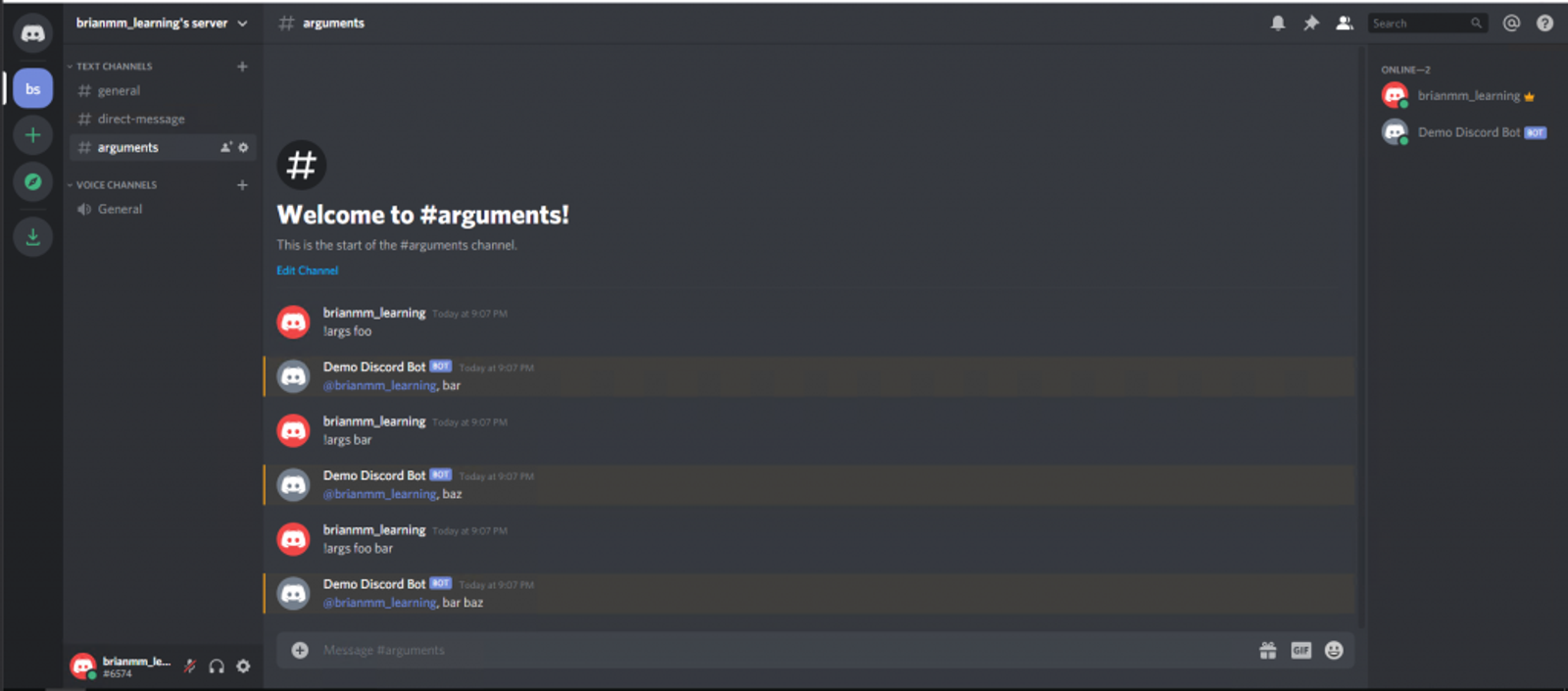
This is how many bots actually work. The bots are coded to use a common prefix (I.e.; !args
) and perform different actions based on the content of the message they receive.
If you are enjoying this content, please feel free to stop by my Discord (fullstack.chat) to hang out with other developers, including me!