Say there are various snippets of code that you’ve built up over some time that could be useful in multiple projects. You COULD copy and paste this code between the projects, but if you find a bug, that means you have to go into each and fix the bug. A better approach would be to create a package that can be shared across multiple projects. That’s exactly what you’ll learn how to do in this article.
To demonstrate this, I’m going to create a simple npm package that exports a command that combines a name with Hello
, so the result of passing in my name would output Hello Brian!
Here’s the code.
const sayHello = function (name) {
console.log("Hello " + name + "!")
}
module.exports = {
sayHello
}
You'll also need to add the following to package.json. Notice what's called the scope at the end of the URL. For your project, replace this with your GitHub username.
"publishConfig": {
"registry":"https://npm.pkg.github.com/@YOUR_USERNAME"
},
Create an empty repository to hold your package. When you do, make sure you tick the Private radio.
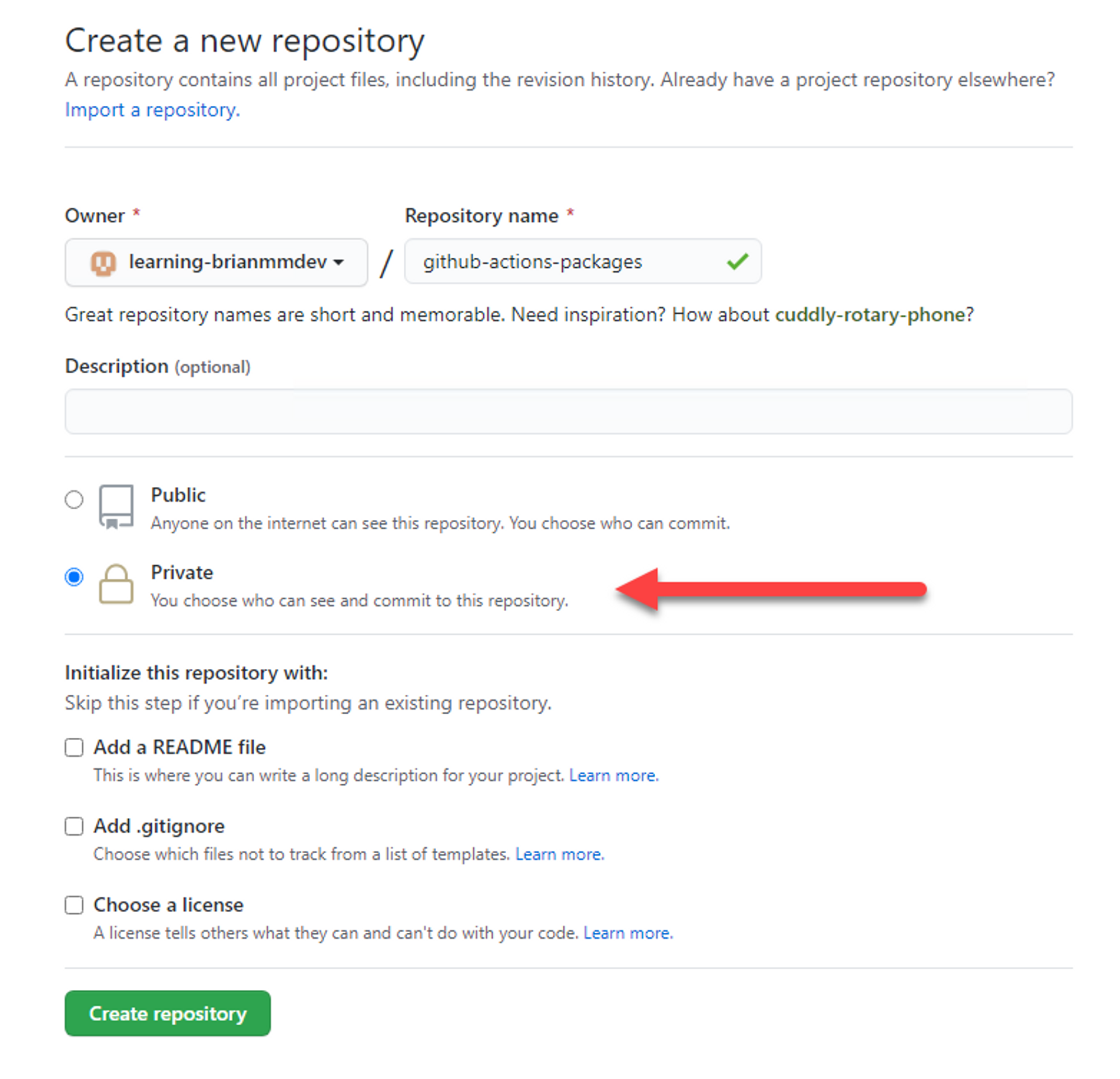
After you've pushed the code to your repo, go into Actions and click "set up a workflow yourself →"
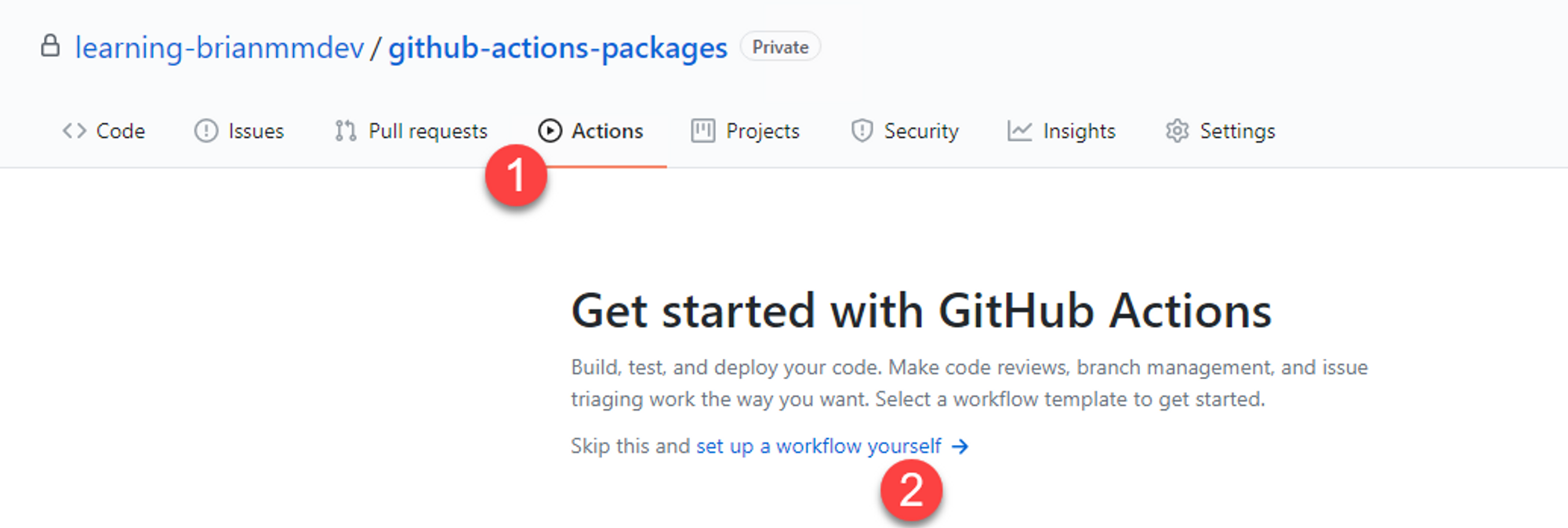
name: Node.js Package
on:
push:
branches:
- master
jobs:
publish-gpr:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- uses: actions/setup-node@v1
with:
node-version: 12
registry-url: https://npm.pkg.github.com/
scope: '@YOUR_USERNAME'
- run: npm install
- run: npm publish
env:
NODE_AUTH_TOKEN: ${{secrets.GITHUB_TOKEN}}
Once done, click Start commit, then Commit new file.
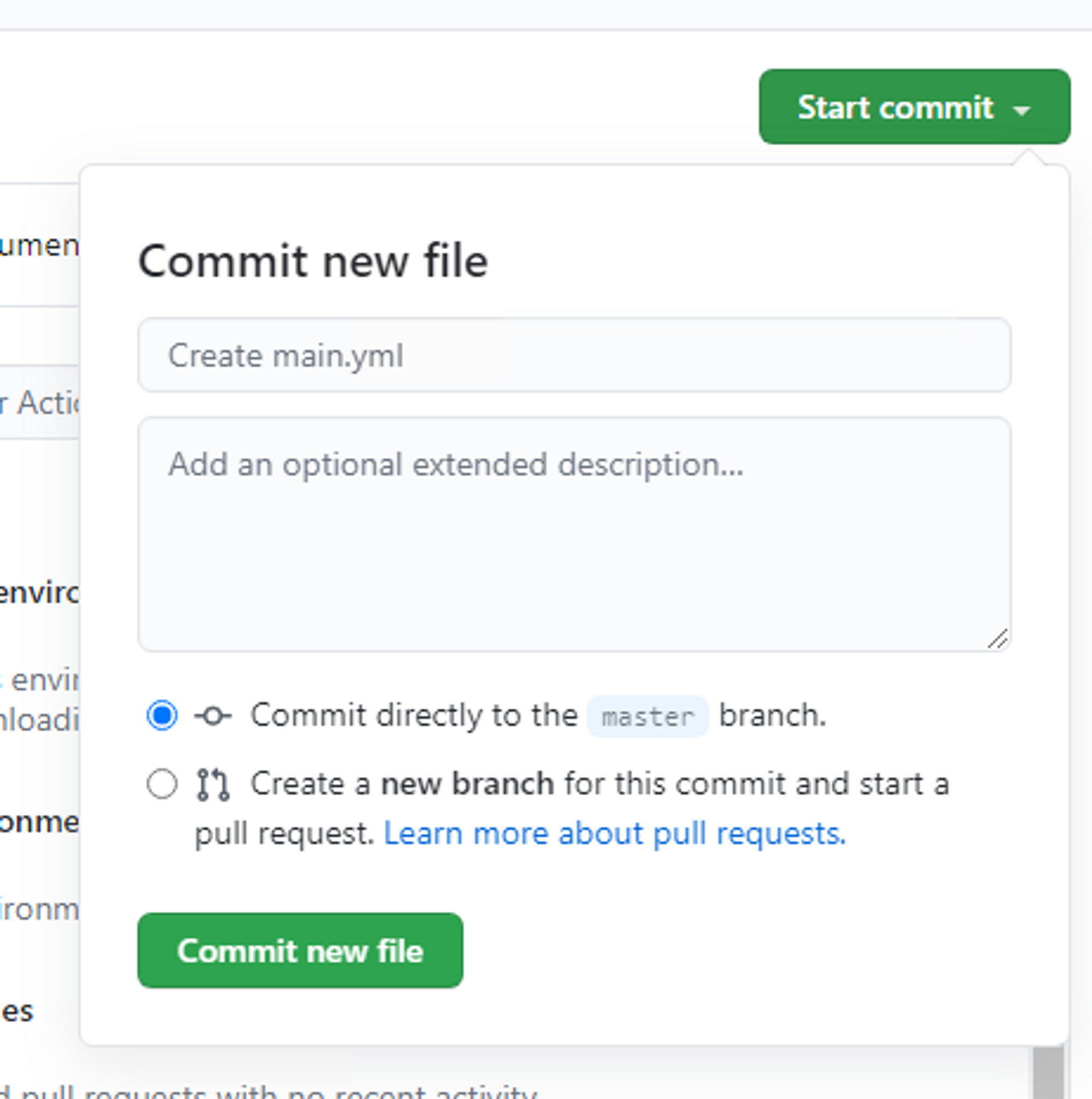
Now head back into Actions and you should see your project building. Once its done and you receive the following, you should be ready to use your package in another project.

You can also see the package details by going back to the repository home and clicking on the package on the right sidebar.
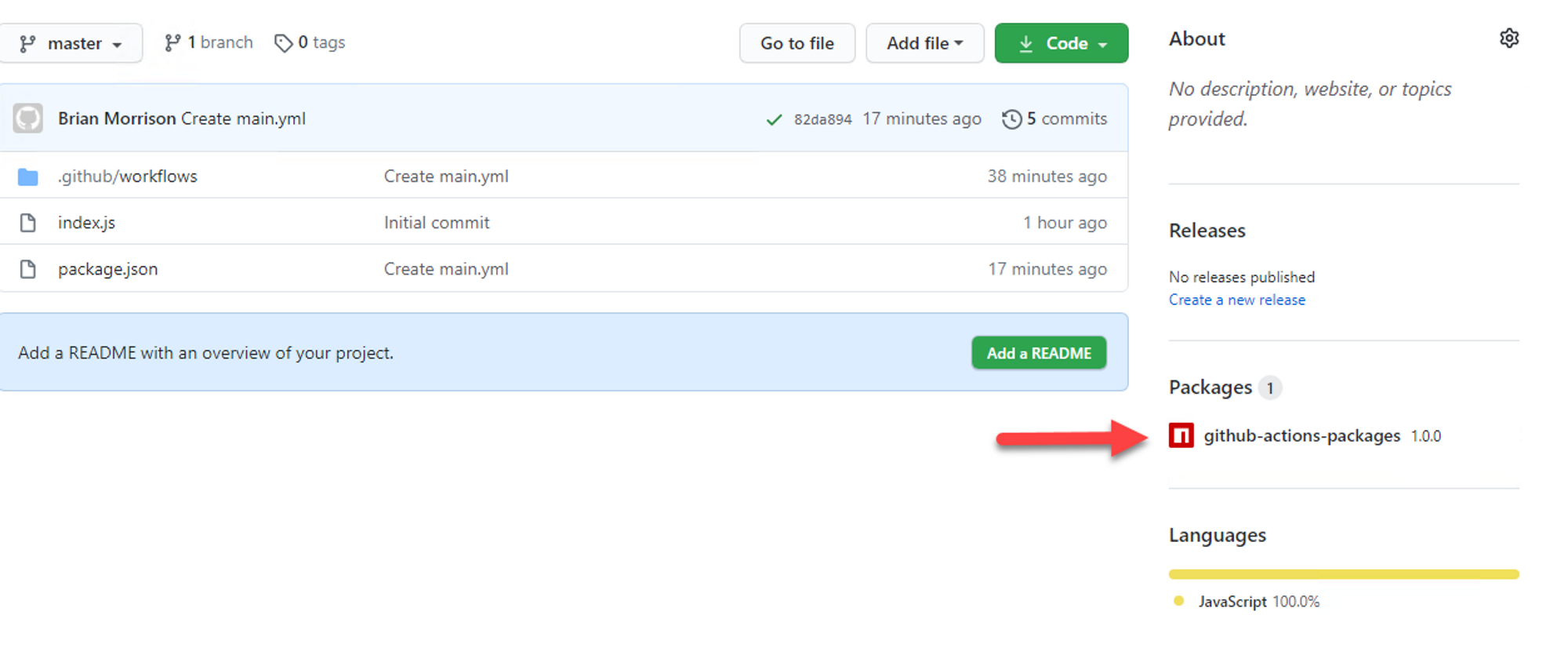
Adding to a Project
Adding to a project is pretty simple. The only thing you'll need to is add a file to the root of your project called .npmrcwith some info telling your local npm where to look for packages for your scope. Before you do, you'll need to generate a personal access token (or PAT) from GitHub that is used to authenticate with private package feeds.
To get your PAT, click on your profile image on the upper right of GitHub and select Settings. Then go to Developer Settings, Personal Access Tokens, and Generate New.
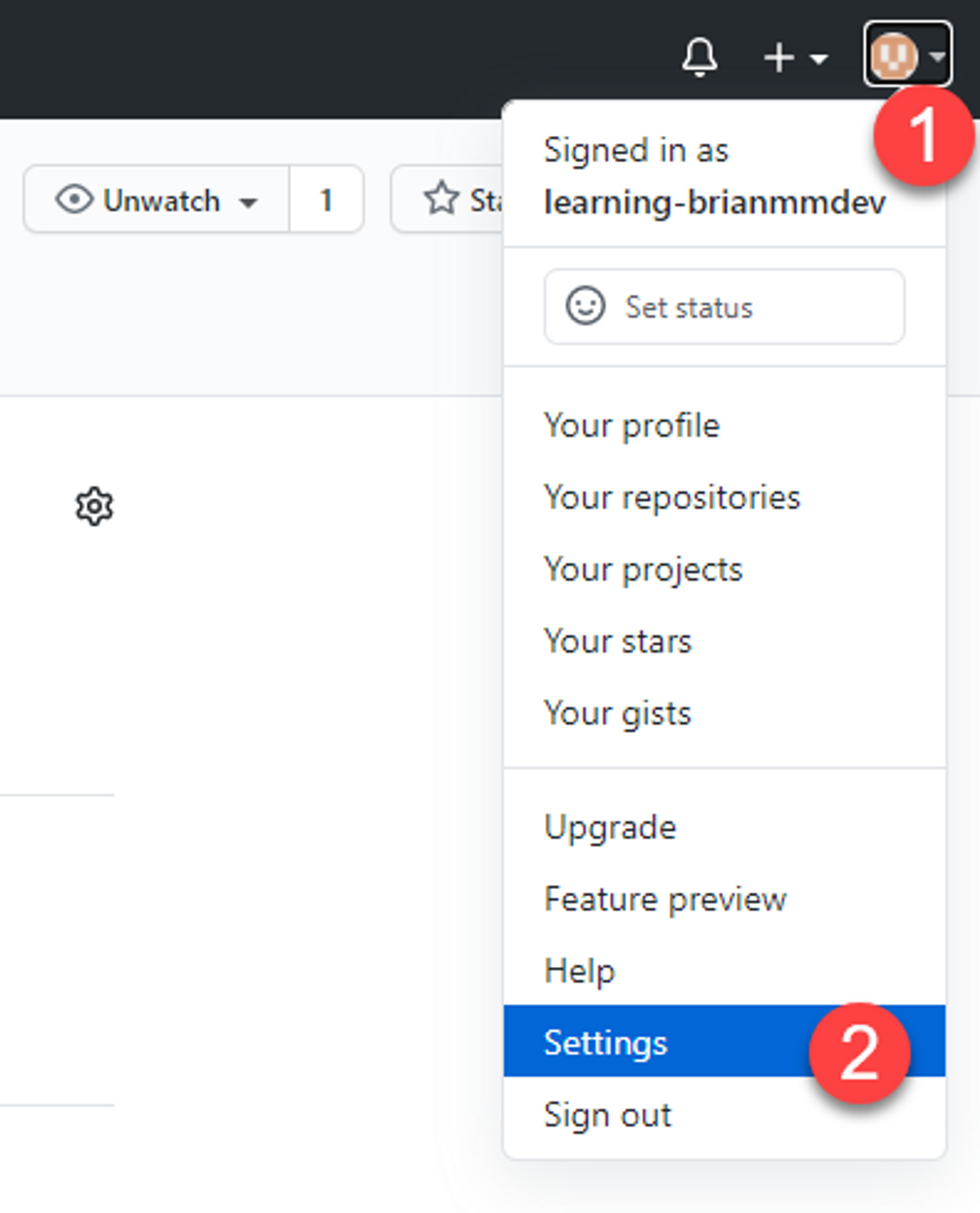
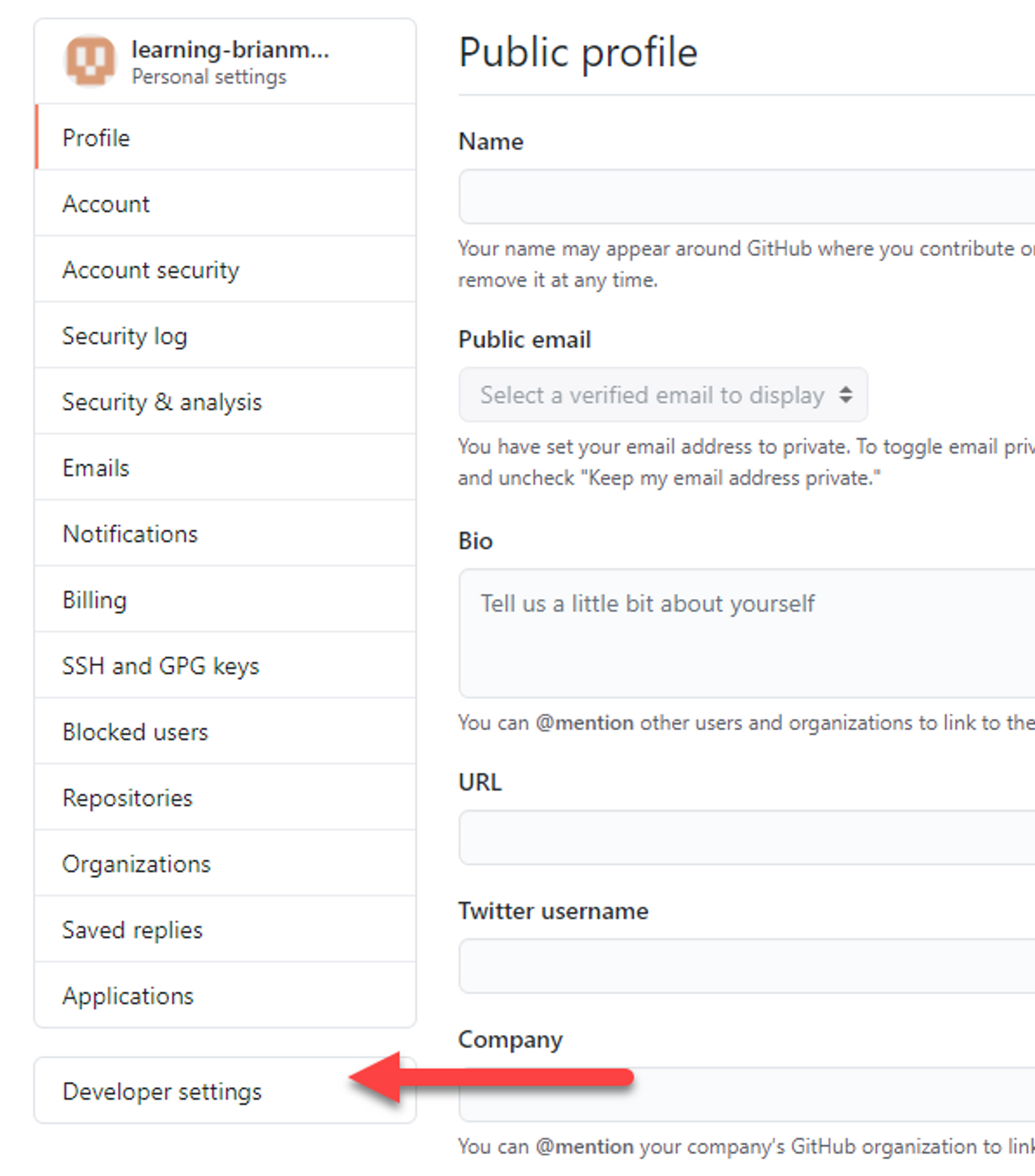
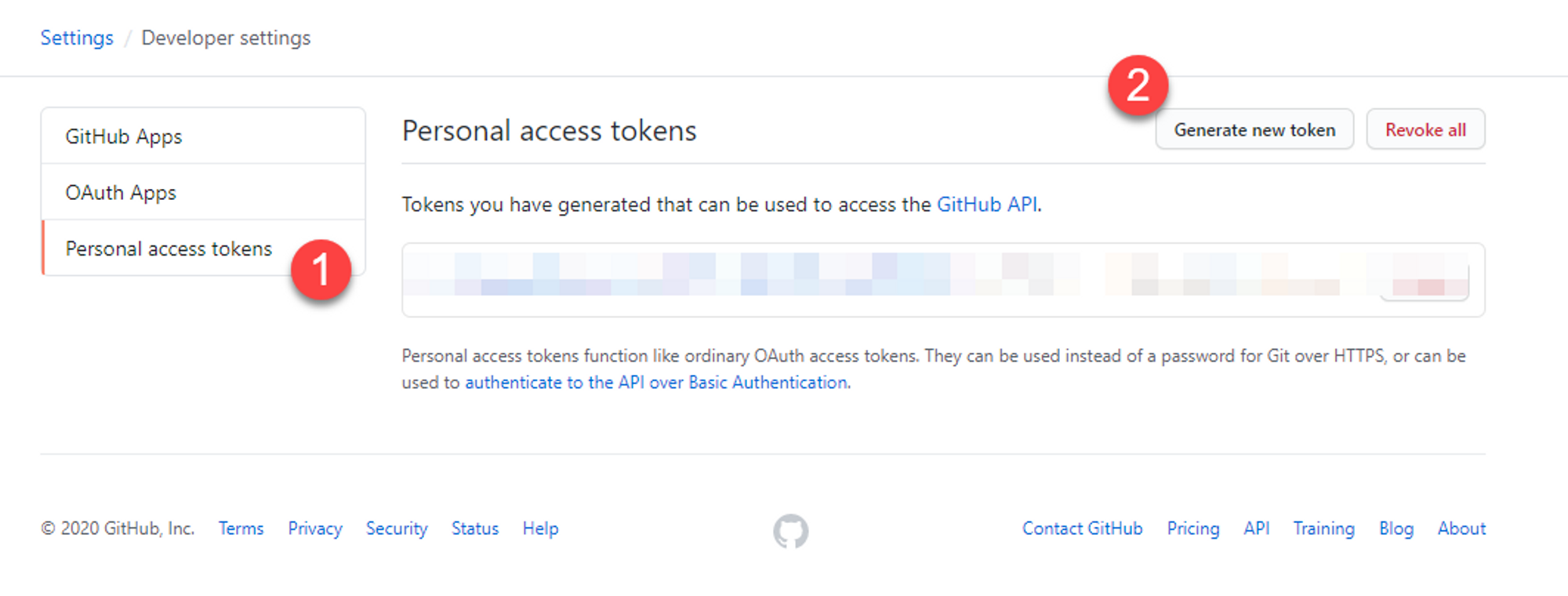
In the next window, give your token a name and select the read:pacakges scope. Make sure not to check any other boxes as this might give the token too much access to your account. Scroll to the bottom and click Generate Token.
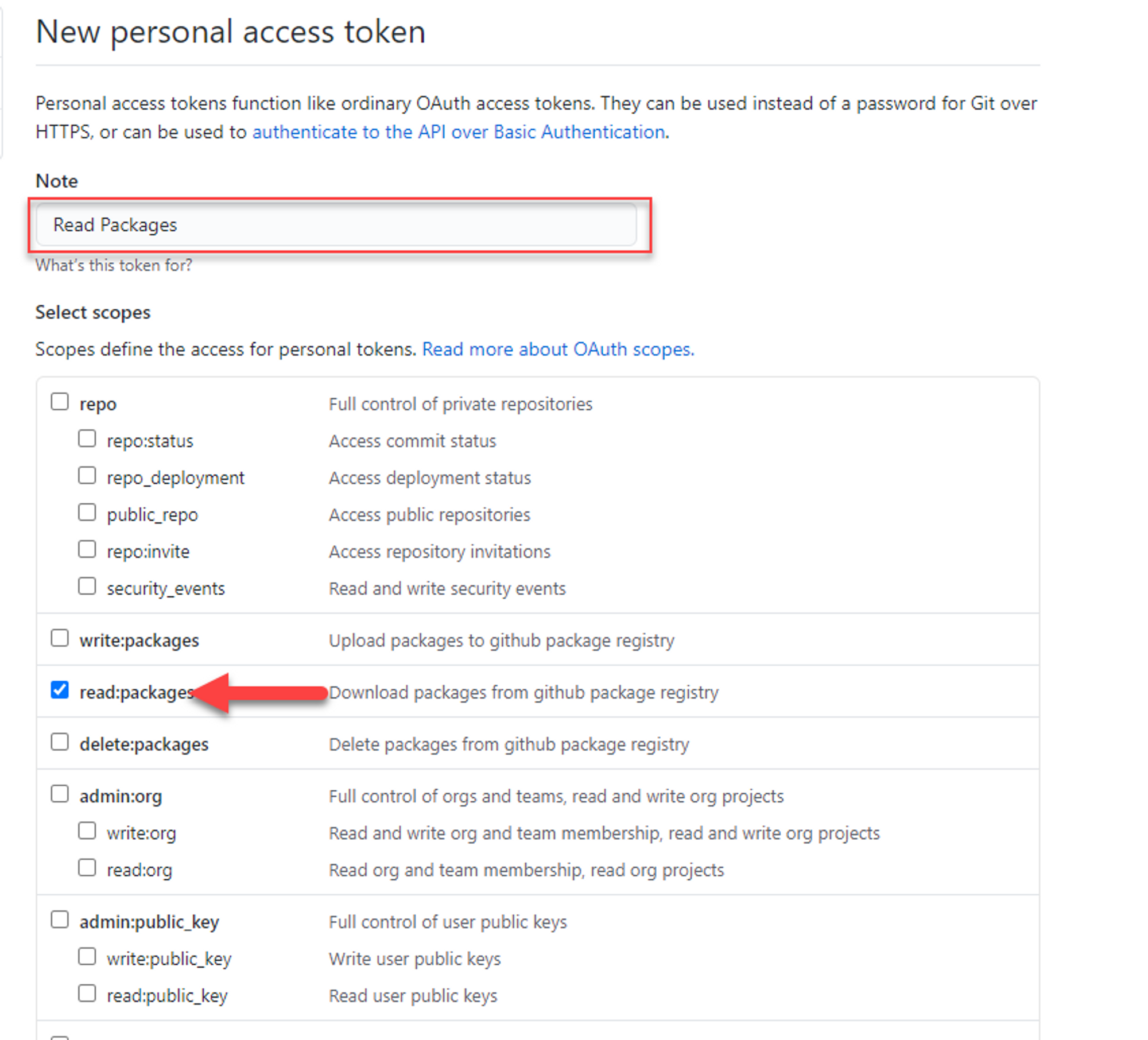
Once you create the token, you'll be put back into the previous view with the PAT listed. You MUST copy it now as you can't get it again.
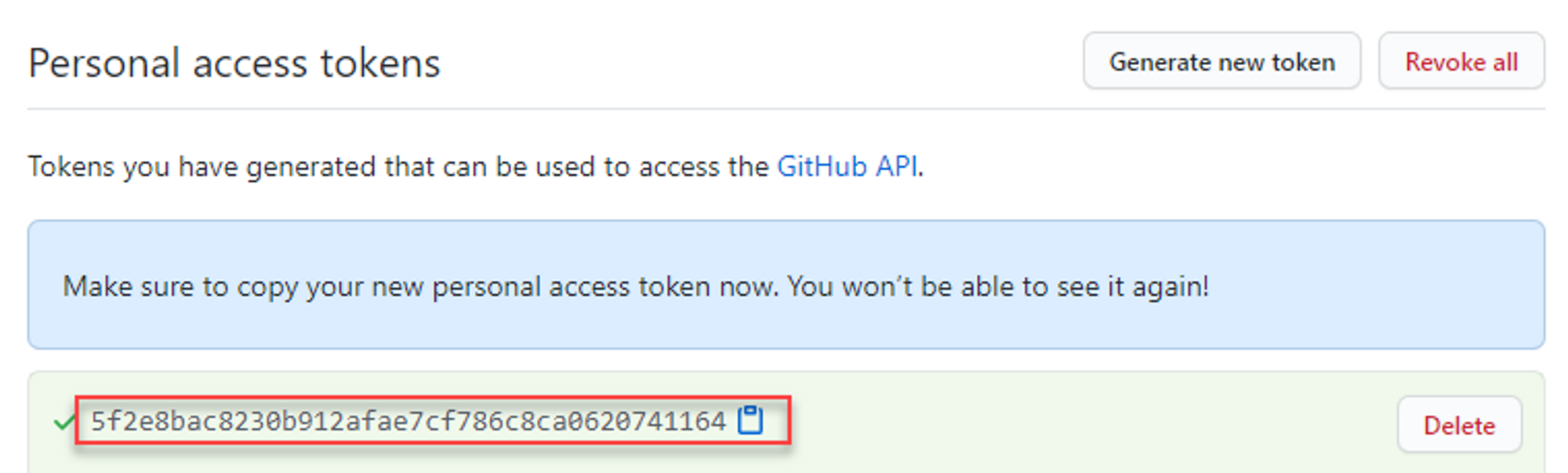
Tokens should be treated as passwords. Never share them with anyone you don't trust. Furthermore, in the following section, I'll be adding the token directly into the repo. This is generally bad practice and a build variable should be used instead (more here). I'm doing this as a demonstration.
Now lets get that .npmrc file setup. Create the file in the project you want to use your new package with and add the following content to it. Replace the scope with your username, and the token with your token.
@YOUR_USERNAME:registry=https://npm.pkg.github.com/
//npm.pkg.github.com/:_authToken=YOUR_TOKEN
Now you can install the package just like you would any other NPM package. Replace the scope & project name with whatever yours is.
npm install @learning-brianmmdev/github-actions-packages
In the project, I created a small file just to test the exported function from the package like so.
let demoPackage = require('@learning-brianmmdev/github-actions-packages')
demoPackage.sayHello('Brian')
And here is the output of testing this.

Congratulations! You now have your very own private package that can be reused across projects you build.