A while back, there was a movement in the tech industry called Chat Ops. Essentially, it was the idea that chat applications (like Discord) can be used to trigger commands that facilitate parts of the Software Development process. In order to do this, bots are used to communicate with other services to trigger actions, gather data, etc.
Today, we’re going to explore how to do this using Axios, my preferred Node HTTP client.
Installing Axios
Axios is an HTTP client library for Node. I prefer it over others basically due to its simplicity in setting up requests. Install Axios by opening your terminal and issuing the command `npm install axios`. This will install the library into our package for the bot to use.
Pulling Gists with Our Bot
An easy way to demonstrate how to interact with external services is using the GitHub api, specifically for Gists. Gists are a way for developers to create public (or private) snippets of code. Not quite full blown repositories, but helpful nonetheless.
We’re going to add a command called `!gists` that will pull in all public Gists for a user and list the links in our channel. Add the following code into the message handler;
const axios = require('axios')
module.exports = {
// Define the prefix
prefix: "!gists",
// Define a function to pass the message to
fn: (msg) => {
let args = msg.content.split(' ')
let username = args[1];
// Check to make sure a username is provided
if (!username) {
msg.reply("Please provide a username.");
} else {
// Setup our Axios options. Perform a GET request to the url specified
let axiosOptions = {
method: "get",
url: `https://api.github.com/users/${username}/gists`
}
// Pass in the options to Axios to make the request
axios(axiosOptions)
.then(response => {
console.log(response.data)
})
}
}
}
Now try to issue the command !gists
in your server. You should have some console output of the data that was returned from the API request;
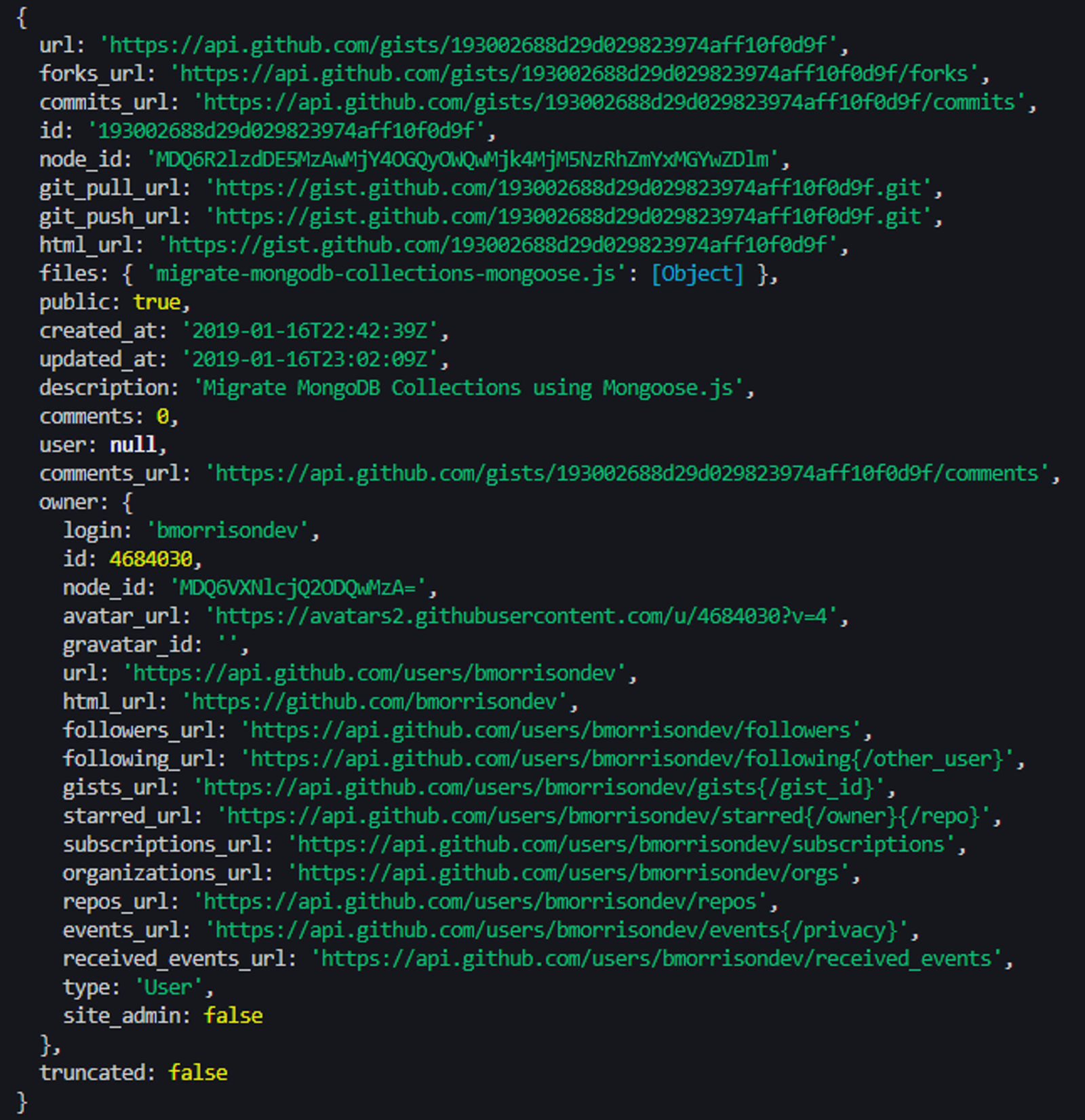
Using this, we can determine the structure of the data and pull what we need from it. For simplicity, I’m going to just loop through the description
and output it to an Embed.
Replace the console.log()
line with this;
// Map our data to grab only the description
let gists = response.data.map(el => el.description)
// Loop through the descriptions and add a line to the embed
let embedDescription = "";
gists.forEach(el => {
embedDescription += `- ${el}\n`
});
// Create the embed & send it to the channel
let embed = new Discord.MessageEmbed()
.setTitle(`Gists for ${username}`)
.setDescription(embedDescription)
.setURL(`https://www.github.com/users/${username}/gists`)
msg.channel.send(embed);
Now when you send the !gists
command in your server, you should see a list of gists that GitHub user has created.
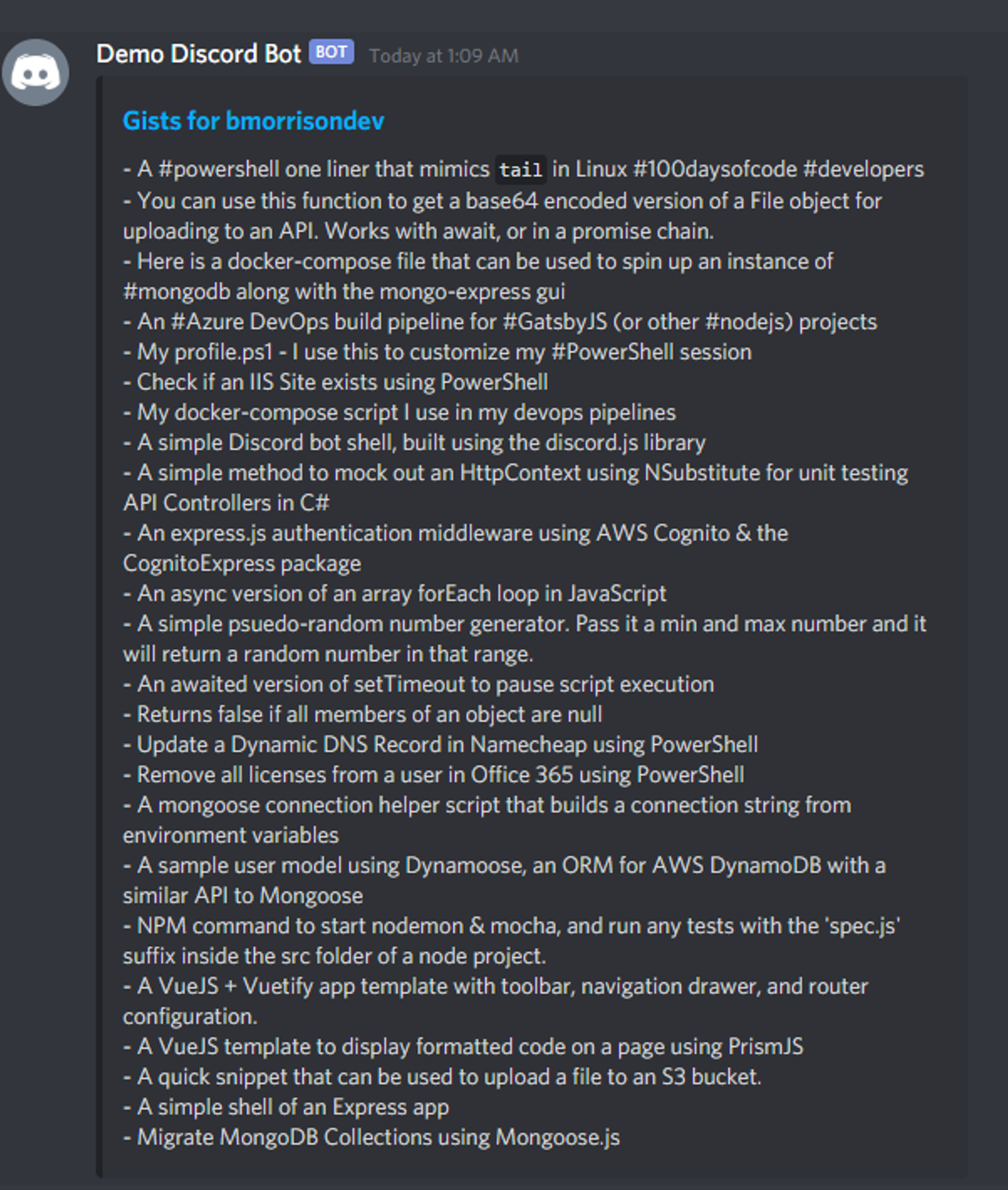
My next article will go over how to use Discord webhooks to receive information from external services and display them in our server.