You ever want to build a command line app with NodeJS to run on systems that might not have NodeJS installed? Then this article is for you. I'll be explaining how to do exactly that with a library called pkg.js. This article was inspired by a CLI utility I created for my work to send notifications to Microsoft Teams from our build servers.
The GitHub link for this article links to a starter repo I built to quickly get up and running with pkg.js.
Prerequisites
Before getting started, make sure you have the following installed;
- VSCode
- NodeJS
Project Setup
We're going to build a simple app that just logs "Hello world!" to the console. Open VSCode, pick a folder for your project, and initialize it by issuing npm init -y
in the terminal. Then create a file called index.js. Inside the file, just write the following;
console.log("Hello world!");
Test the app by running the following in the terminal.
node app.js
Provided you get the following output, everything is setup as expected.
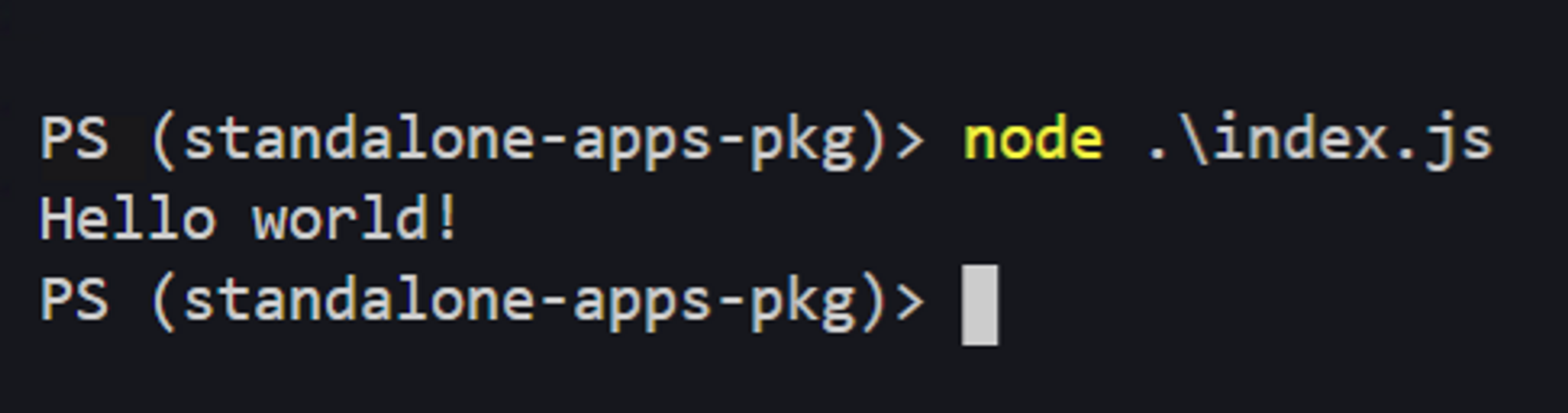
Now lets get pkg setup. Run the following in the terminal to install the library globally.
npm install -g pkg
Once the install is finished, head into package.json and add a node like so;
"bin": "index.js",
What we're doing is telling the pkg library where to look for its entry point.
Building Binaries
By default, pkg will create binaries for Windows, Mac, and Linux. You can create these by issuing the following command in the terminal;
pkg .
Once finished, you should see three binaries in the root if your project;
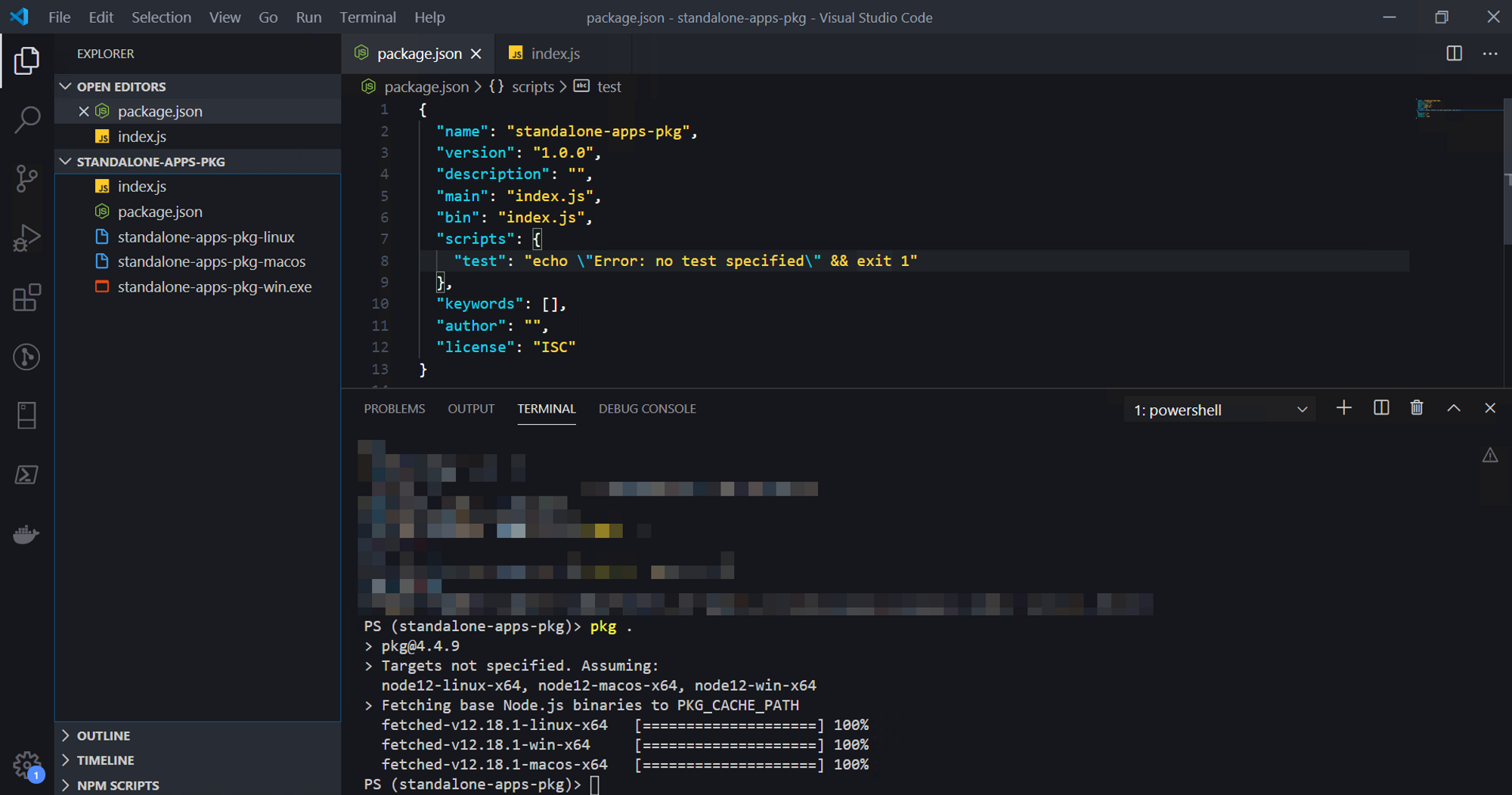
I'm on windows, so I can run it by typing .\standalone-apps-pkg-win.exe
in the terminal. Lets make sure we get Hello world! again;

Handling Arguments
You can actually pass in arguments into your app as well like so;
.\myapp.exe --name='Brian'
Which is exactly what we'll do now. Lets update index.js to look like so;
// Find the argument we're looking for
let name = process.argv.find(el => el.startsWith('--name='))
// Get rid of the name of the argument
name = name.replace('--name=', '')
// Log the output
console.log("Hello " + name + "!")
Now build your app again using pkg .
and pass in the name argument like shown above (feel free to use your name 😉);

The binaries built should be able to run on any system, regardless if Node is installed or not!