Trello is an extremely popular Kanban tool that can easily be used to manage a variety of things, from projects to idea boards. One of the most powerful features though is the API, which can be used to modify pretty much anything within Trello. In this article, we're going to touch on some of the different things you can do with the Trello API such as creating & updating cards, but the concepts are exactly the same across all endpoints. Lets dive in.
Before following along, install Postman if you haven't already. We'll be using this to test a few calls to the API.
Exploring the Docs
Trello actually has three different APIs. The one we will be using is the Rest API, which is documented at https://developer.atlassian.com/cloud/trello/rest/api-group-actions/. If you continue developing with Trello, this is a link you'll want to keep handy as it outlines every possible way you can use their API.
Getting Your API Credentials
In order to authenticate against the Trello API, we'll need to create a Trello App so that we can get an API Token & Key. Head over to https://trello.com/app-key, acknowledge the terms if prompted, and lick Show API Key. You should see a page like this. Save your Key off somewhere since we will need it for all API requests.
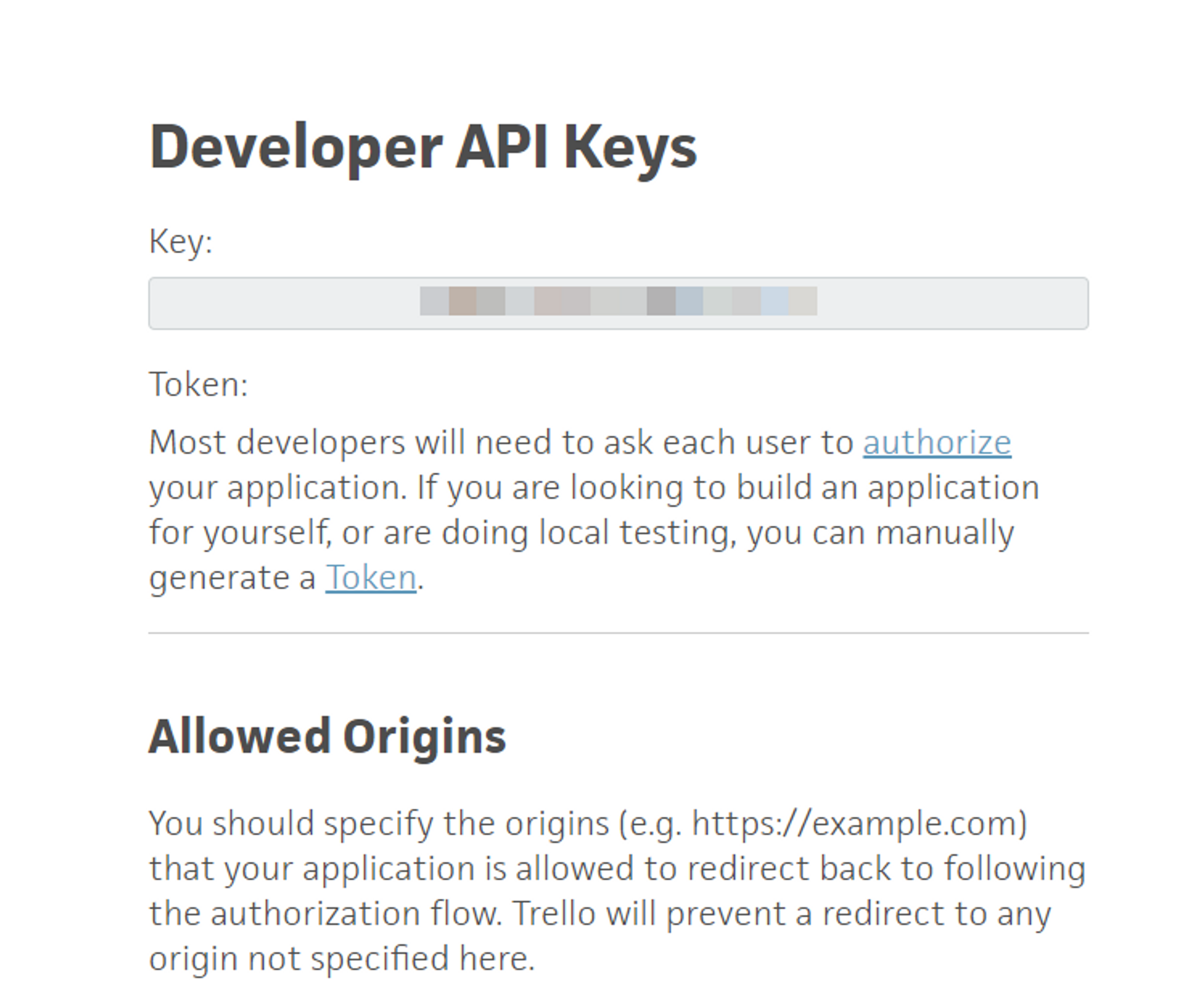
Next click on Token. You should be redirected to a page saying that Server Token (or something similar) is requesting access to your account. Scroll to the bottom & click Allow. You'll be provided a Token that is required for requests as well.
Anyone who has your token can access your account, so treat it like a password!
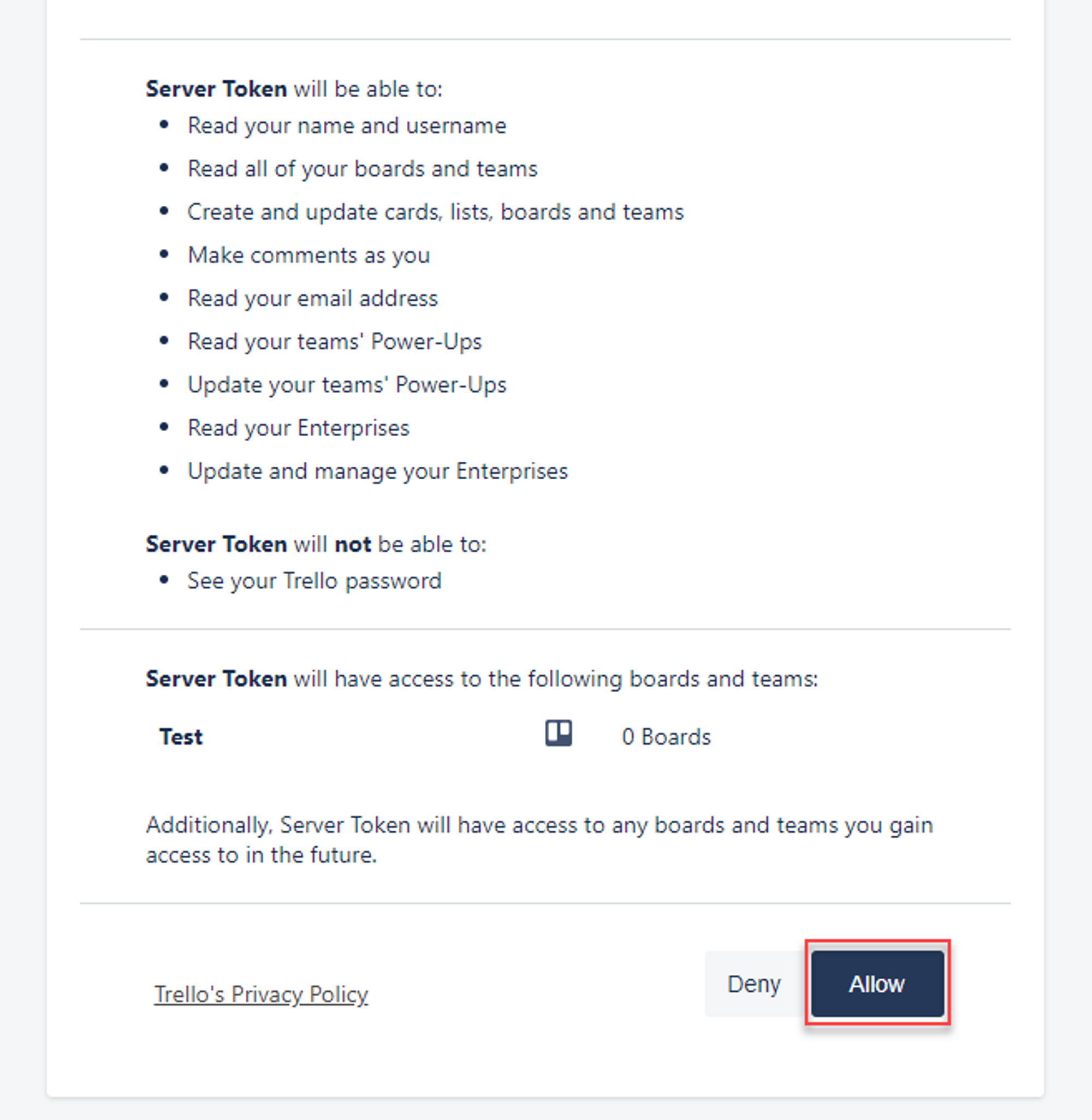
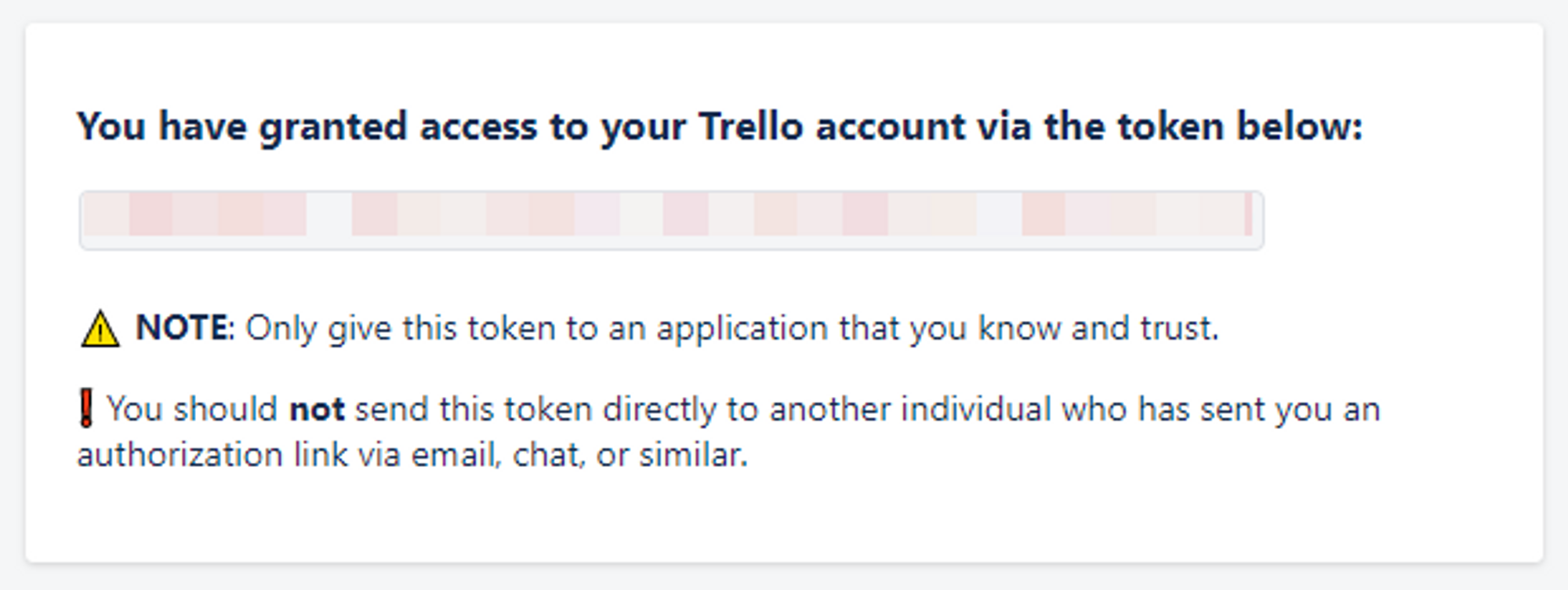
API Basics
Trello is a bit different than other APIs in the way they receive data. Most calls will not leverage the HTTP body and when you send data, you'll send it as query parameters in the URL like so.
https://api.trello.com/1/boards/{idBoard}?key={yourKey}&token={yourToken}
While scanning the docs, you'll notice the endpoints are displayed without a domain. To use them, just add [https://api.trello.com](https://api.trello.com)
in front of them and you should be good to go.
Another thing worth noting is that all calls will require the key
& token
to be sent. This is what authenticates you to Trello, your request will be denied otherwise. Lets test a few API calls now.
Testing the API
In Trello, Cards are pretty much the base entity of the system. Anything that is tracked in Trello is done via Cards, so we're going to test a few things around Cards. Lets take a look at the URL you can use to create a card;
POST https://api.trello.com/1/cards?key={yourKey}&token={yourToken}&name={cardName}&idList={idList}
Besides the token
and key
params, the only other field technically required for this call is idList
(which as you can assume, is the unique ID of the list you want the card to be added to), but the card isn't going to be very useful if you don't at least provide the name
field as well.
To find the Id of any list or board, you could use those APIs, but an easier method is to export a board to JSON to see all of the embedded data for that board (which will include lists, cards, etc). To do this, open the board menu, select More, Print and Export, and finally Export as JSON. If you are using Chrome with the JSON Formatter extension, it should just display the JSON in a nicely formatted document that you can navigate to pull out the info you are interested in.
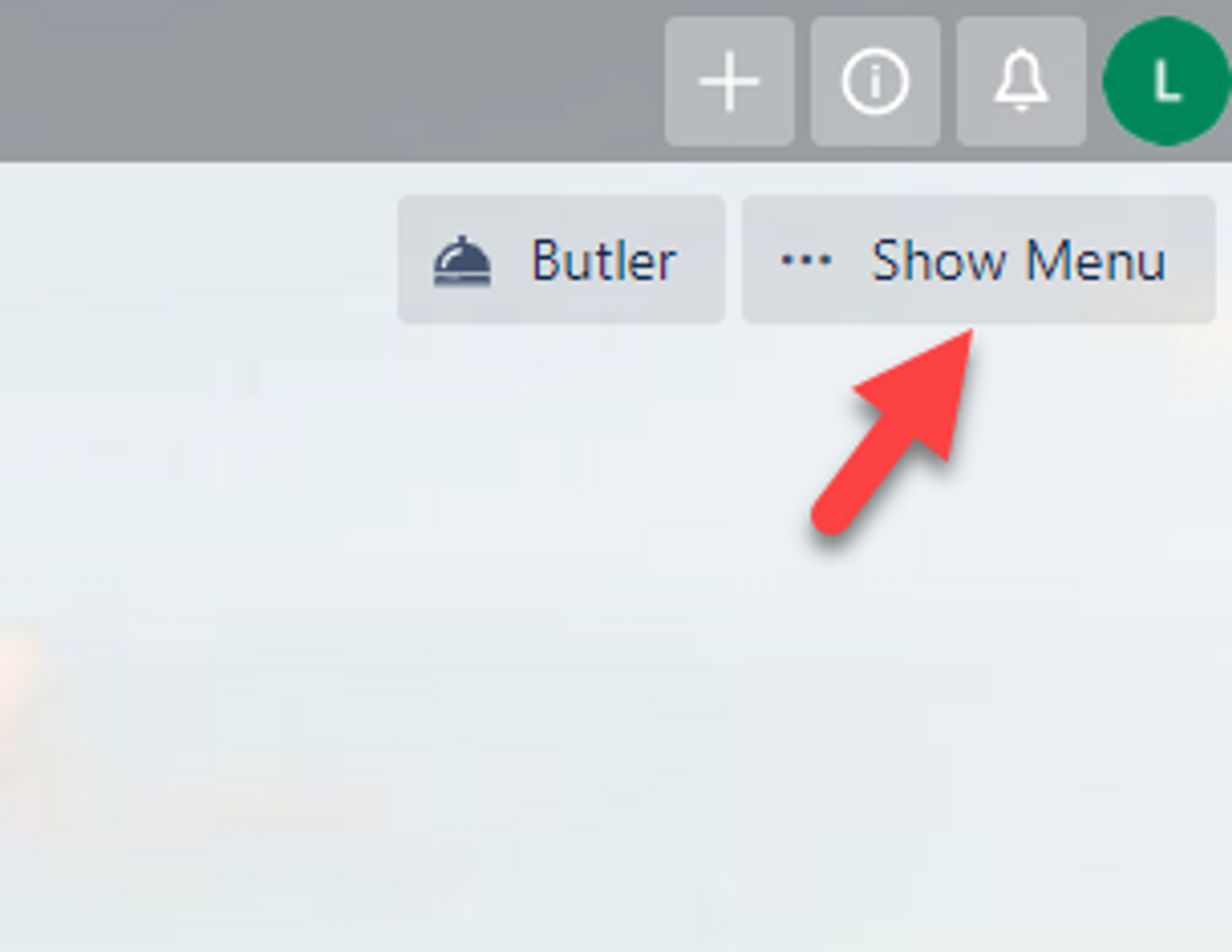
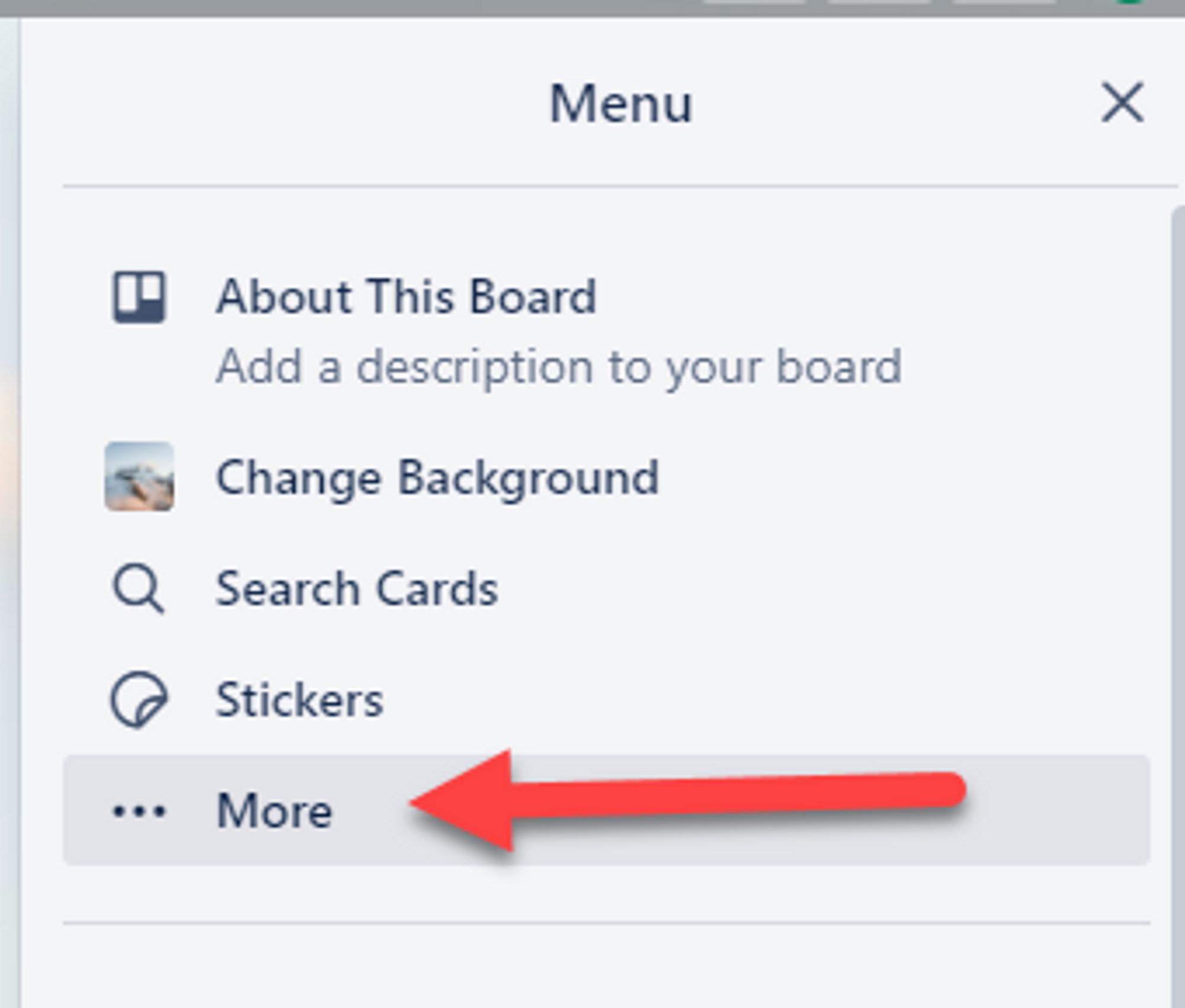
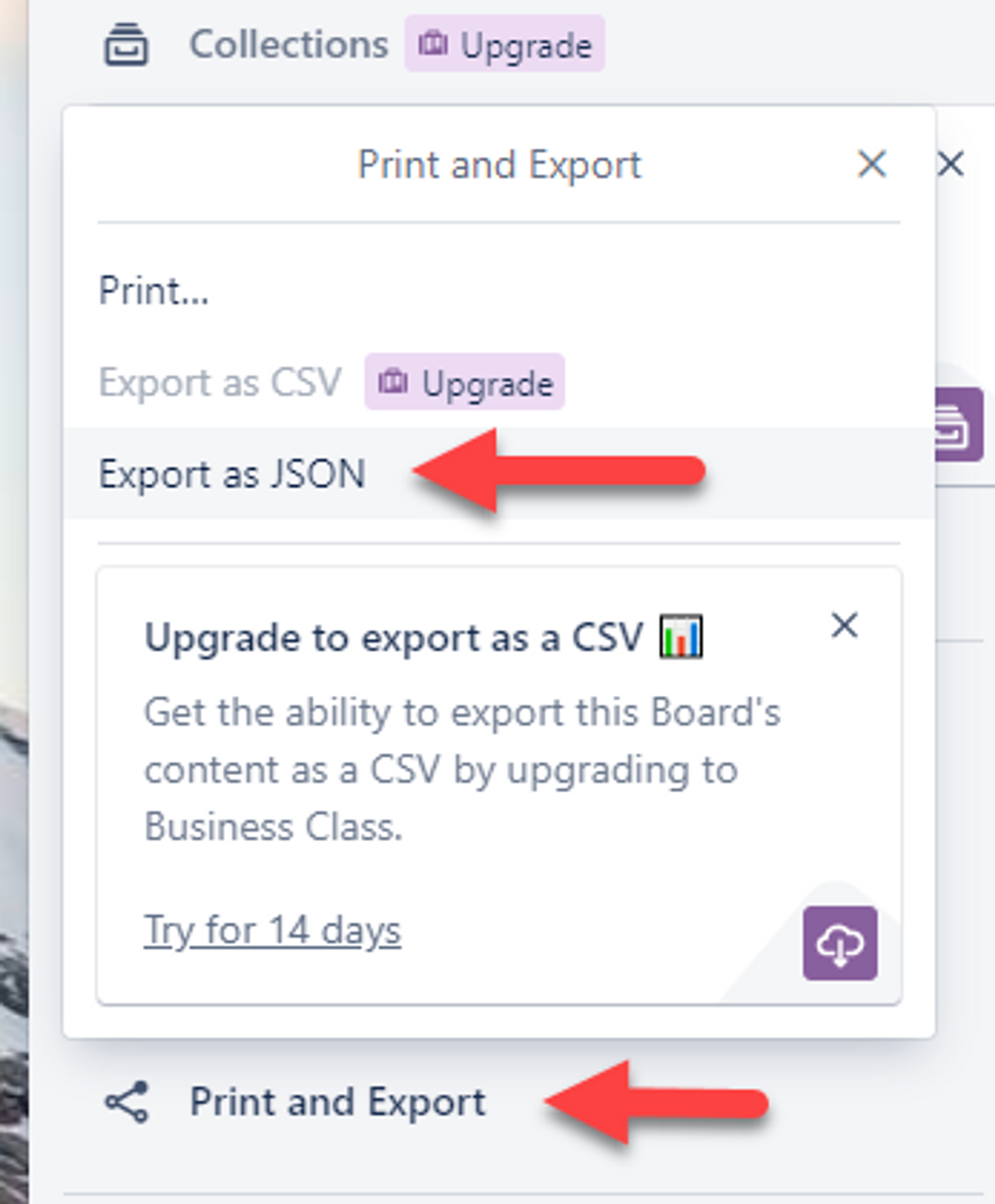
Now if you scroll down to the bottom, you can see an array of lists. In the starter board, there are already a couple lists, so I'm going to grab the Id of the To Do list.
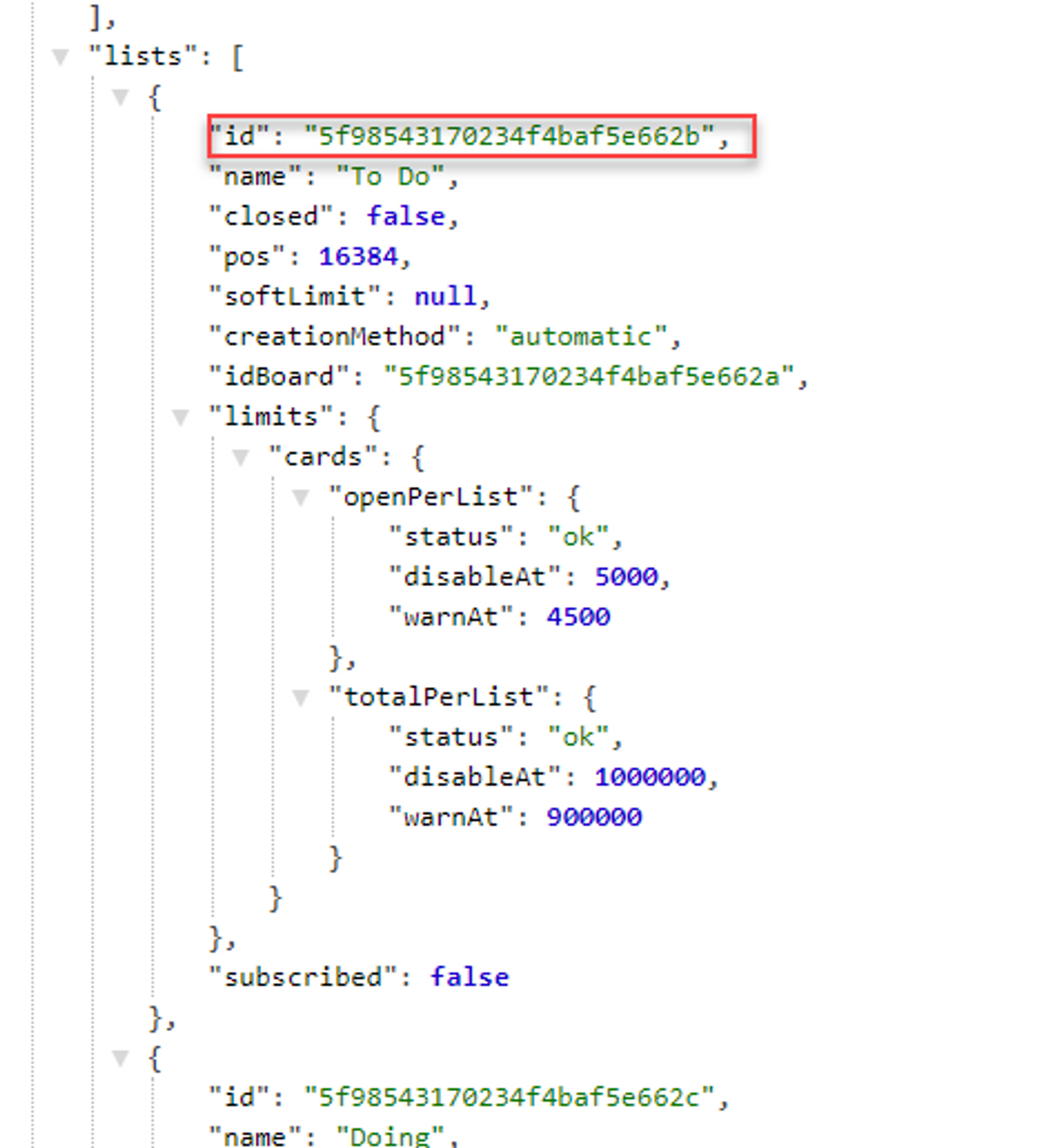
So using Postman, I can now create a new card in this list by submitting a POST to the following URL. Note that any data sent in the URL must be properly encoded for Trello to recognize it (which is why the name is My%20new%20card
instead of My new card
).
https://api.trello.com/1/cards?key={yourKey}&token={yourToken}&name=My%20new%20card&idList=5f98543170234f4baf5e662b
Trello will respond with the data about the new card that was created if it was successful.
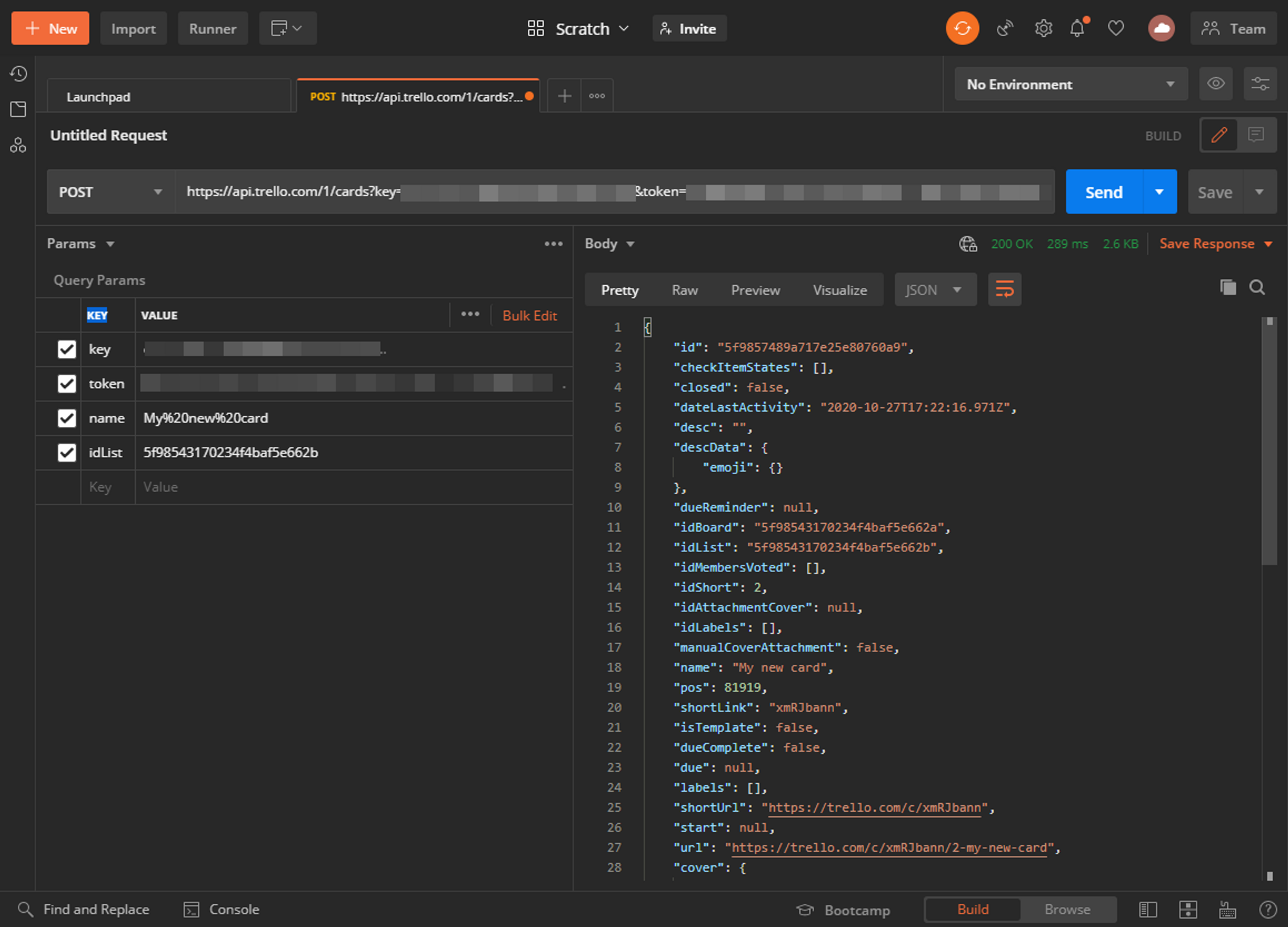
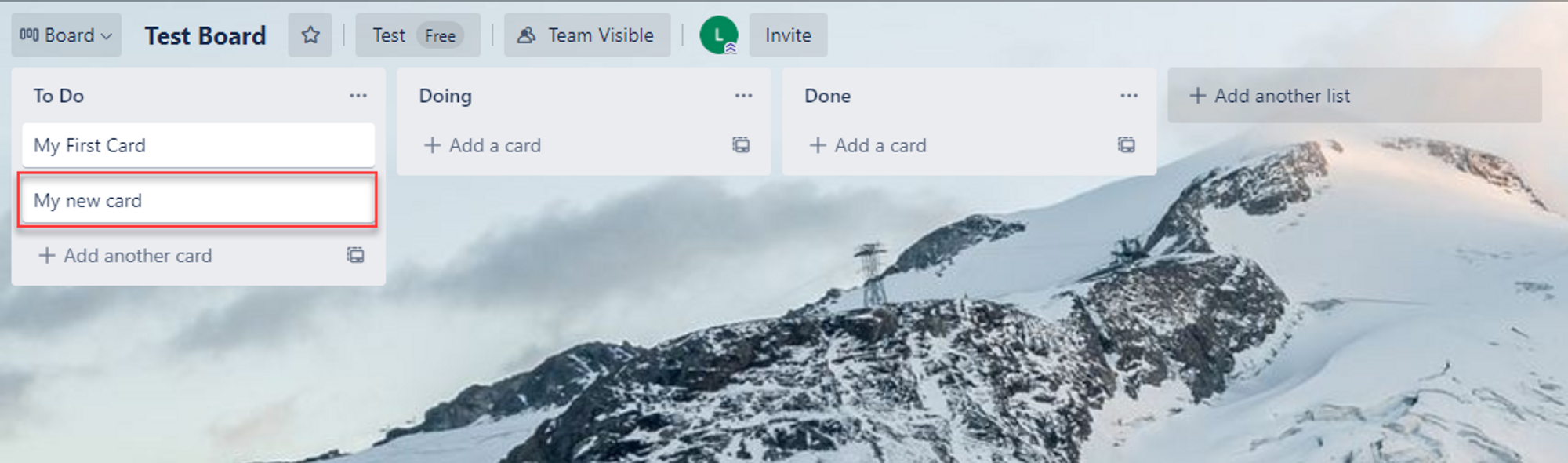
Now lets say we have started working on our new card and we want to move it to the Doing list. To do this, we'll send a PUT request to the cards endpoint with the Id of the card at the end of the url, like so. (I used the same Export function as above to get the cards Id, and the Id of the list I want to send it to)
https://api.trello.com/1/cards/5f9857489a717e25e80760a9?key={yourKey}&token={yourToken}&idList=5f98543170234f4baf5e662c
As you can see we still receive the card data back, but if we check the board, it has moved to the next column.
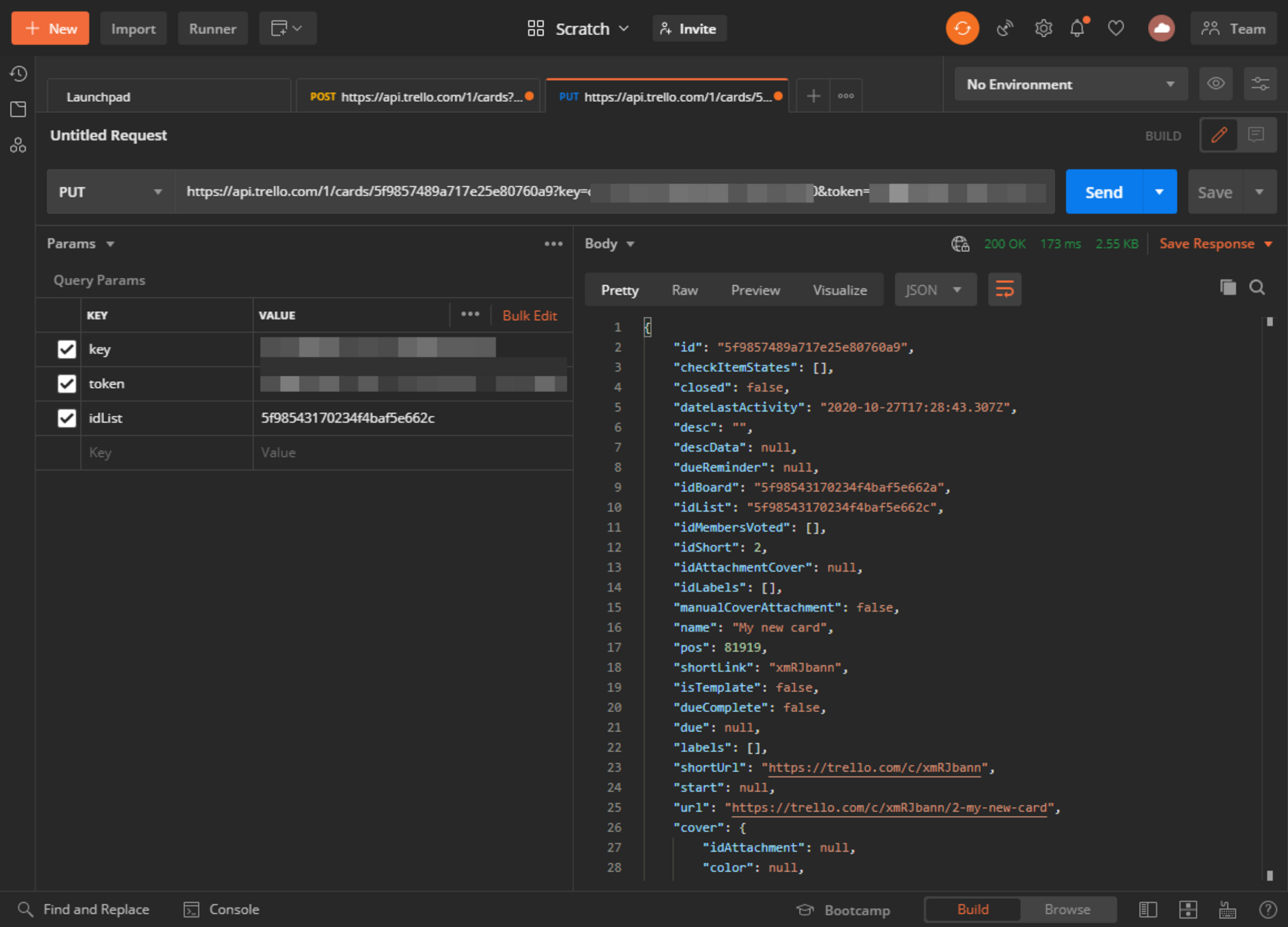
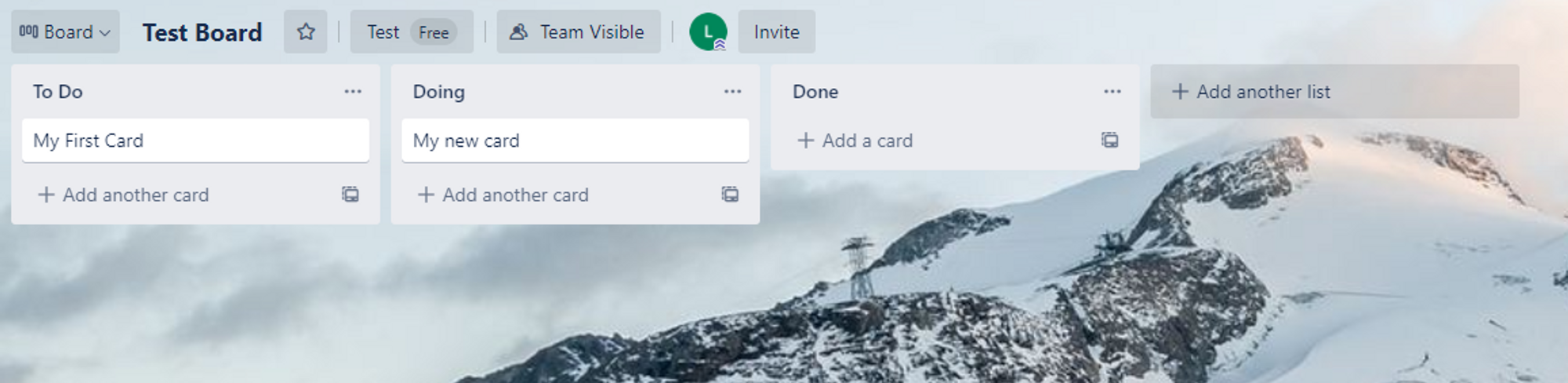
Whats Next
Once you understand some of the basics of working with the Trello REST API, those concepts translate across all endpoints and concepts in the API. At this point, its just a matter of deciding what you want to create or modify, finding the endpoint definition in the docs, and writing your HTTP requests to what you need it to.
In the next article in this series, I'll explore how to create and use webhooks and have Trello automatically notify an external system when certain events happen.